郊狼优化算法python
时间: 2023-10-20 18:09:14 浏览: 249
郊狼优化算法(WLO)是一种基于自然界中狼群捕猎行为的启发式优化算法。它模拟了狼群中的领袖、追随者和孤狼之间的相互作用,通过不断地迭代搜索最优解。以下是一个简单的Python实现:
```python
import random
import numpy as np
def WLO(obj_func, lb, ub, dim, SearchAgents_no, Max_iter):
Alpha_pos = np.zeros(dim)
Alpha_score = float("inf")
Beta_pos = np.zeros(dim)
Beta_score = float("inf")
Delta_pos = np.zeros(dim)
Delta_score = float("inf")
Positions = np.zeros((SearchAgents_no, dim))
for i in range(dim):
Positions[:, i] = np.random.uniform(0, 1, SearchAgents_no) * (ub[i] - lb[i]) + lb[i]
for l in range(0, Max_iter):
for i in range(0, SearchAgents_no):
fitness = obj_func(Positions[i, :])
if fitness < Alpha_score:
Alpha_score = fitness
Alpha_pos = Positions[i, :]
if fitness > Alpha_score and fitness < Beta_score:
Beta_score = fitness
Beta_pos = Positions[i, :]
if fitness > Alpha_score and fitness > Beta_score and fitness < Delta_score:
Delta_score = fitness
Delta_pos = Positions[i, :]
a = 2 - 2 * l / Max_iter
for i in range(0, SearchAgents_no):
r1 = random.random()
r2 = random.random()
A = 2 * a * r1 - a
C = 2 * r2
b = 1
l1 = random.random()
p = random.random()
if p < 0.5:
if abs(A) < 1:
D_alpha = abs(C * Alpha_pos - Positions[i, :])
X1 = Alpha_pos - A * D_alpha
else:
rand_leader_index = np.floor(SearchAgents_no * random.random())
X_rand = Positions[int(rand_leader_index), :]
D_X_rand = abs(C * X_rand - Positions[i, :])
X1 = X_rand - A * D_X_rand
else:
distance2Leader = abs(Alpha_pos - Positions[i, :])
# Eq. (2.5)
X1 = distance2Leader * np.exp(b * l1) * np.cos(2 * np.pi * l1) + Alpha_pos
r1 = random.random()
r2 = random.random()
A = 2 * a * r1 - a
C = 2 * r2
b = 1
l1 = random.random()
p = random.random()
if p < 0.5:
if abs(A) < 1:
D_beta = abs(C * Beta_pos - Positions[i, :])
X2 = Beta_pos - A * D_beta
else:
rand_leader_index = np.floor(SearchAgents_no * random.random())
X_rand = Positions[int(rand_leader_index), :]
D_X_rand = abs(C * X_rand - Positions[i, :])
X2 = X_rand - A * D_X_rand
else:
distance2Leader = abs(Beta_pos - Positions[i, :])
# Eq. (2.5)
X2 = distance2Leader * np.exp(b * l1) * np.cos(2 * np.pi * l1) + Beta_pos
r1 = random.random()
r2 = random.random()
A = 2 * a * r1 - a
C = 2 * r2
b = 1
l1 = random.random()
p = random.random()
if p < 0.5:
if abs(A) < 1:
D_delta = abs(C * Delta_pos - Positions[i, :])
X3 = Delta_pos - A * D_delta
else:
rand_leader_index = np.floor(SearchAgents_no * random.random())
X_rand = Positions[int(rand_leader_index), :]
D_X_rand = abs(C * X_rand - Positions[i, :])
X3 = X_rand - A * D_X_rand
else:
distance2Leader = abs(Delta_pos - Positions[i, :])
# Eq. (2.5)
X3 = distance2Leader * np.exp(b * l1) * np.cos(2 * np.pi * l1) + Delta_pos
Positions[i, :] = (X1 + X2 + X3) / 3
lb = np.tile(lb, (SearchAgents_no, 1))
ub = np.tile(ub, (SearchAgents_no, 1))
Positions = np.clip(Positions, lb, ub)
return Alpha_pos, Alpha_score
# 示例函数
def sphere(x):
return sum(x ** 2)
# 测试
lb = [-100] * 30
ub = [100] * 30
WLO(sphere, lb, ub, 30, 50, 500)
```
阅读全文
相关推荐
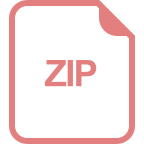
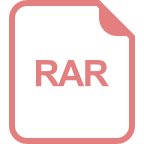
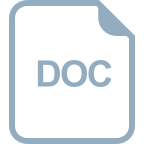

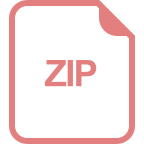
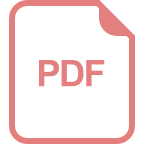
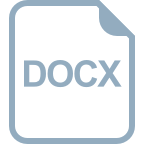
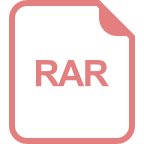
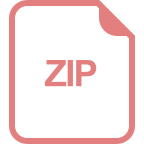
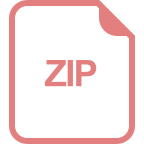
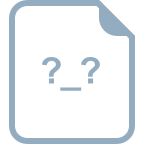
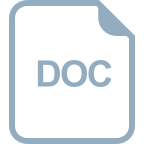
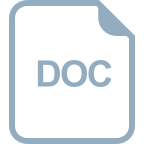
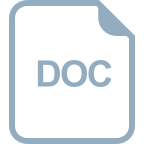
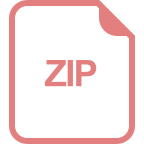