tensorflow1.14不用keras进行多GPU联合训练
时间: 2024-10-24 09:13:56 浏览: 15
在TensorFlow 1.14版本中,虽然官方Keras库(keras)已经与TensorFlow紧密集成,但直接使用`tensorflow.keras`进行多GPU联合训练可能不如使用`tf.distribute.Strategy`那样直观。然而,如果你确实需要在TF 1.14环境下实现多GPU训练,可以采用以下方法[^2]:
1. **设置环境变量**:
```python
os.environ['CUDA_VISIBLE_DEVICES'] = '0,1,2,3,4,5' # 指定要使用的GPU ID
```
2. **创建单GPU模型**:
```python
single_model = build_model()
single_model.load_weights(path, by_name=True)
```
3. **转换为多GPU模型**:
```python
from tensorflow.keras.utils import multi_gpu_model
gpus = len(gpu_list.split(',')) # 获取GPU数量
model = multi_gpu_model(single_model, gpus=gpus)
```
请注意,这种方法是在较低层次上操作,对于更高级别的API如`model.fit()`,你可能需要手动编写并调用分布式训练循环。由于这是在较旧版本的TensorFlow中,可能会有兼容性限制。
相关问题
我的整个基于tensorflow1.14的模型已经搭建完毕且能正常运行,现在想能使其多GPU设备同时训练,用keras是不是不可行
Keras通常是在TensorFlow之上构建的高级API,对于多GPU并行训练的支持相对直接和简单。在TensorFlow 1.x版本中,你可以通过`tf.distribute.Strategy`来实现跨GPU的分布式训练。虽然Keras本身不直接支持多GPU训练(在1.x版本中),但你可以使用`MirroredStrategy`或`MultiWorkerMirroredStrategy`等策略来扩展到多个GPU。
下面是一个基本的示例,展示如何在Keras中启用多GPU训练[^1]:
```python
# 导入必要的模块
import tensorflow as tf
# 检查当前可用的GPU数量
gpus = tf.config.list_physical_devices('GPU')
if gpus:
strategy = tf.distribute.MirroredStrategy(gpus=gpus)
else:
print("No GPUs available")
# 将模型和优化器置于策略作用域内
with strategy.scope():
model = ... # 定义你的模型
optimizer = ... # 定义优化器
# 创建一个数据分布器
dataset = ...
data_iterator = iter(dataset)
# 开始训练
for epoch in range(num_epochs):
for step, (x, y) in enumerate(data_iterator):
with tf.GradientTape() as tape:
predictions = model(x, training=True)
loss_value = compute_loss(y, predictions)
gradients = tape.gradient(loss_value, model.trainable_variables)
optimizer.apply_gradients(zip(gradients, model.trainable_variables))
```
注意,这只是一个基础示例,实际操作可能需要调整以适应你的具体模型结构和数据加载方式。另外,在切换到多GPU时,务必考虑同步问题以及潜在的性能瓶颈。
tensorflow-gpu和keras版本对应
### 回答1:
TensorFlow-GPU和Keras版本对应如下:
TensorFlow-GPU 1.13.1对应Keras 2.2.4
TensorFlow-GPU 1.14.对应Keras 2.3.
TensorFlow-GPU 2..对应Keras 2.3.1
TensorFlow-GPU 2.1.对应Keras 2.3.1
TensorFlow-GPU 2.2.对应Keras 2.4.
注意:以上版本仅供参考,具体版本对应关系可能会因为不同的环境和配置而有所不同。建议在使用时查看官方文档或者参考其他可靠来源。
### 回答2:
TensorFlow-GPU是深度学习框架TensorFlow的加速版本,它使用GPU加速模型的训练和推理,可以提高训练速度和模型性能。而Keras是一种用于构建神经网络的高级API,它可以运行在多个深度学习框架之上,包括TensorFlow。
TensorFlow-GPU和Keras的版本需要对应才能正常运行。具体而言,如果您使用的是TensorFlow-GPU 1.13,则您需要使用Keras 2.2.4,使用TensorFlow-GPU 2.0时需要使用Keras 2.3.1版本。如果您安装的版本不对应,这可能会导致您的代码无法运行或产生意料之外的结果。
在安装TensorFlow-GPU和Keras时,最好使用Anaconda、pip或conda等软件包管理器来安装,这样可以方便地安装对应版本的包。同时,在安装之前,建议先查看文档和官方网站,了解所使用的TensorFlow-GPU和Keras版本对应的详细信息。
总之,正确安装TensorFlow-GPU和Keras的版本对应是保证深度学习模型顺利训练的前提,需要认真对待。
### 回答3:
TensorFlow是一款流行的深度学习框架,它被广泛使用于各种机器学习和深度学习任务中。TensorFlow GPU(tensorflow-gpu)是TensorFlow的GPU版本,它通过利用图形处理器(GPU)的并行计算能力来加速深度学习模型的训练和推理速度。而Keras是一个高级的深度学习框架,它可以被用来构建复杂的神经网络模型。
TensorFlow GPU和Keras都有不同的版本,而这些版本通常需要互相兼容才能顺利工作。以下是TensorFlow GPU和Keras版本对应的一些常见规则:
1. TensorFlow 1.x系列与Keras 2.x系列对应:TensorFlow 1.x系列是通过Session API来管理计算图和计算资源的,而Keras 2.x系列是作为一个高级API来运行在TensorFlow 1.x系列上的。因此,TensorFlow 1.x版本的用户应该使用Keras 2.x版本。
2. TensorFlow 2.x系列自带Keras API:TensorFlow 2.x系列的版本中已经内置了Keras API,因此,TensorFlow 2.x的用户应该使用内置的Keras API,而不是使用外部的Keras。
3. TensorFlow 2.x系列中的Keras API具有向后兼容性:由于TensorFlow 2.x中的Keras API具有向后兼容性,因此,用户可以在TensorFlow 2.x版本中使用旧版的Keras模型。
4. 安装TensorFlow GPU时需要注意版本号:在安装TensorFlow GPU时,需要注意与Keras版本的兼容问题。对于TensorFlow 1.x,建议安装与Keras 2.x兼容的版本;对于TensorFlow 2.x,建议使用内置的Keras API。
总之,TensorFlow GPU和Keras版本之间的兼容性非常重要,用户在使用这两个框架时,应该仔细检查其版本号,并确保版本之间的兼容性。否则,可能导致运行时错误和不可预测的问题。
阅读全文
相关推荐
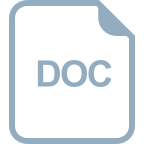
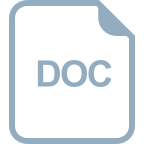
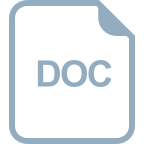
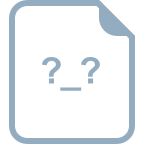
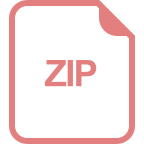
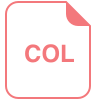
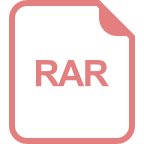
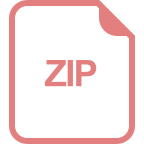
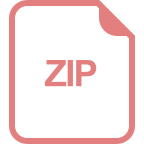
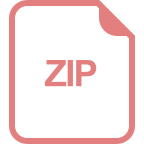
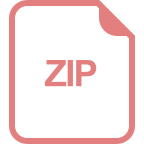
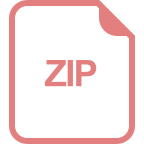
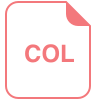
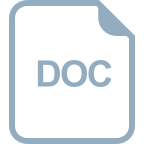
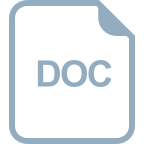
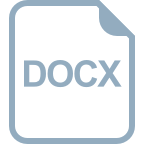