请写一段可以实现输入一个整数,在链表中查找这个数,找到第一个后将该节点删除的c++方法。
时间: 2024-11-20 22:44:24 浏览: 14
为了在链表中查找一个整数并删除第一个出现的节点,你需要创建一个链表结构,然后遍历链表进行搜索。以下是一个简单的C++实现,使用`std::list`(因为它支持常量时间的O(1)插入和删除操作):
```cpp
#include <iostream>
#include <list>
// 定义链表节点结构体
struct ListNode {
int value;
ListNode* next;
};
// 创建新节点
ListNode* createNode(int val) {
ListNode* newNode = new ListNode();
newNode->value = val;
newNode->next = nullptr;
return newNode;
}
// 查找并删除给定值的第一个节点
ListNode* deleteFirst Occurrence(std::list<ListNode*>& list, int target) {
ListNode* current = list.begin(); // 遍历链表
for (ListNode* node = current; node != list.end(); ++node) {
if (node->value == target) { // 如果找到目标节点
list.erase(current); // 删除当前节点
break; // 找到后就退出循环
}
current = node->next; // 移动到下一个节点
}
return list.empty() ? nullptr : list.front(); // 返回新的链表头(可能为空)
}
// 示例:创建链表、添加元素、查找并删除元素
int main() {
std::list<ListNode*> myList;
myList.push_back(createNode(10));
myList.push_back(createNode(20));
myList.push_back(createNode(30));
myList.push_back(createNode(10)); // 添加第二个10
ListNode* result = deleteFirstOccurrence(myList, 10);
if (result) {
std::cout << "Deleted element: " << result->value << std::endl;
} else {
std::cout << "Element not found" << std::endl;
}
return 0;
}
```
在这个示例中,`deleteFirstOccurrence`函数会在链表中查找第一个值为`target`的节点,如果找到了就会删除它,并返回新的链表头节点。如果没有找到,它会返回`nullptr`。
阅读全文
相关推荐
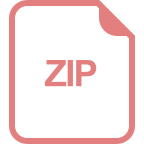
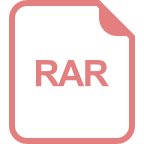
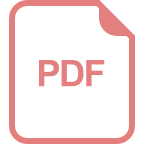




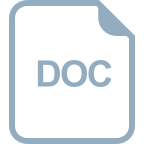
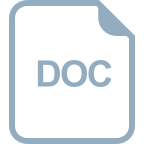









