center loss tensorflow
时间: 2023-09-18 15:13:47 浏览: 91
中心损失(center loss)是一种用于人脸识别和人脸验证任务的损失函数。它的目标是学习一个特征空间,使得同一类别的样本在该空间中尽可能接近一个中心点,而不同类别的样本则远离这个中心点。
在TensorFlow中实现center loss可以遵循以下步骤:
1. 定义输入:首先,定义输入的占位符或张量,包括输入图像数据和标签。
```python
input_images = tf.placeholder(tf.float32, [None, 224, 224, 3], name='input_images')
input_labels = tf.placeholder(tf.int64, [None], name='input_labels')
```
2. 定义特征提取网络:使用一个卷积神经网络(例如ResNet或VGG)来提取图像特征。
```python
# 定义卷积神经网络模型
def conv_net(input_images):
# 假设使用ResNet作为特征提取网络
# ...
return features
# 提取图像特征
features = conv_net(input_images)
```
3. 定义中心点和中心损失:为每个类别定义一个中心点,并计算样本与其对应中心点的欧氏距离。
```python
# 获取类别数量
num_classes = 10
# 初始化中心点矩阵
centers = tf.get_variable('centers', [num_classes, num_features], dtype=tf.float32,
initializer=tf.constant_initializer(0), trainable=False)
# 计算样本与中心点的欧氏距离
distances = tf.reduce_sum(tf.square(features - tf.gather(centers, input_labels)), axis=1)
```
4. 定义中心损失的计算方式:使用欧氏距离计算样本与中心点之间的损失,并更新中心点的位置。
```python
# 定义中心损失
center_loss = tf.reduce_mean(distances)
# 更新中心点
unique_labels, unique_idx, unique_count = tf.unique_with_counts(input_labels)
appear_times = tf.gather(unique_count, unique_idx)
diff = features - tf.gather(centers, input_labels)
diff_expand_dims = tf.expand_dims(diff, 1)
centers_update_op = tf.scatter_sub(centers, input_labels, learning_rate * diff_expand_dims / tf.cast((1 + appear_times), tf.float32))
```
5. 定义总体损失:将中心损失和分类损失(例如交叉熵损失)相结合得到总体损失。
```python
# 定义分类损失(例如交叉熵)
cross_entropy_loss = tf.reduce_mean(tf.nn.sparse_softmax_cross_entropy_with_logits(labels=input_labels, logits=logits))
# 定义总体损失
total_loss = cross_entropy_loss + alpha * center_loss
```
6. 进行模型训练:使用优化器最小化总体损失来更新模型的参数。
```python
# 使用优化器最小化总体损失
optimizer = tf.train.AdamOptimizer(learning_rate)
train_op = optimizer.minimize(total_loss)
```
以上就是使用TensorFlow实现中心损失的基本步骤。在训练过程中,你可以通过运行`centers_update_op`来更新中心点的位置,从而将类别间的距离最小化。
相关推荐
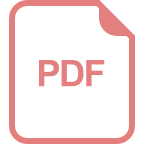















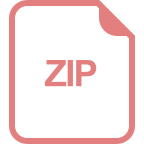
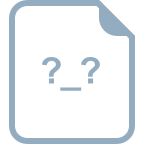