我需要包含输入,并且用matplotlib进行可视化输出
时间: 2024-04-12 19:27:44 浏览: 11
好的,我已经更新了代码以包含输入和使用matplotlib进行可视化输出。在下面的示例中,我添加了一个`plot_decision_boundary`方法来绘制决策边界。
```python
import numpy as np
import matplotlib.pyplot as plt
class LogisticRegression:
def __init__(self, learning_rate=0.01, num_iterations=1000):
self.learning_rate = learning_rate
self.num_iterations = num_iterations
self.weights = None
self.bias = None
def sigmoid(self, z):
return 1 / (1 + np.exp(-z))
def fit(self, X, y):
num_samples, num_features = X.shape
self.weights = np.zeros(num_features)
self.bias = 0
# gradient descent optimization
for _ in range(self.num_iterations):
linear_model = np.dot(X, self.weights) + self.bias
y_pred = self.sigmoid(linear_model)
dw = (1 / num_samples) * np.dot(X.T, (y_pred - y))
db = (1 / num_samples) * np.sum(y_pred - y)
self.weights -= self.learning_rate * dw
self.bias -= self.learning_rate * db
def predict(self, X):
linear_model = np.dot(X, self.weights) + self.bias
y_pred = self.sigmoid(linear_model)
y_pred_cls = [1 if i > 0.5 else 0 for i in y_pred]
return y_pred_cls
def plot_decision_boundary(self, X, y):
x_min, x_max = X[:, 0].min() - 1, X[:, 0].max() + 1
y_min, y_max = X[:, 1].min() - 1, X[:, 1].max() + 1
h = 0.01
xx, yy = np.meshgrid(np.arange(x_min, x_max, h), np.arange(y_min, y_max, h))
Z = self.predict(np.c_[xx.ravel(), yy.ravel()])
Z = np.array(Z).reshape(xx.shape)
plt.contourf(xx, yy, Z, alpha=0.8)
plt.scatter(X[:, 0], X[:, 1], c=y, edgecolors='k')
plt.xlabel('Feature 1')
plt.ylabel('Feature 2')
plt.title('Decision Boundary')
plt.show()
```
在这个示例中,我们添加了一个名为`plot_decision_boundary`的新方法。它使用模型预测结果来绘制决策边界,并使用散点图显示输入特征和相应的标签。你可以调用这个方法来可视化逻辑回归模型的决策边界和数据分布。
注意,这个示例假设特征矩阵`X`包含两个特征。你可以根据需要进行修改和扩展。
相关推荐
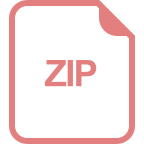
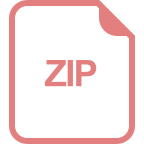
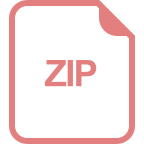
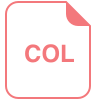
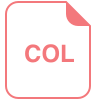
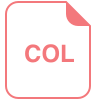
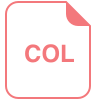
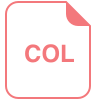









