基于pytorch实现对自定义网络任意层的特征进行tsne可视化
时间: 2023-07-12 07:15:31 浏览: 112
好的,下面是实现的步骤:
1. 首先需要定义自己的网络模型,并加载预训练好的权重。
2. 导入需要用到的库,包括pytorch、numpy、matplotlib等。
3. 定义一个函数来获取任意层的特征。该函数需要输入一个图片和目标层的名称,然后输出该层的特征。
4. 定义一个函数来进行tsne可视化。该函数需要输入一个特征矩阵和标签,然后输出可视化结果。
下面是代码示例:
```python
import torch
import numpy as np
import matplotlib.pyplot as plt
from sklearn.manifold import TSNE
# 定义自己的网络模型
class MyNet(torch.nn.Module):
def __init__(self):
super(MyNet, self).__init__()
self.conv1 = torch.nn.Conv2d(3, 64, 3, padding=1)
self.conv2 = torch.nn.Conv2d(64, 128, 3, padding=1)
self.conv3 = torch.nn.Conv2d(128, 256, 3, padding=1)
self.fc1 = torch.nn.Linear(256*8*8, 1024)
self.fc2 = torch.nn.Linear(1024, 10)
def forward(self, x):
x = torch.nn.functional.relu(self.conv1(x))
x = torch.nn.functional.relu(self.conv2(x))
x = torch.nn.functional.relu(self.conv3(x))
x = x.view(-1, 256*8*8)
x = torch.nn.functional.relu(self.fc1(x))
x = self.fc2(x)
return x
# 加载预训练好的权重
model = MyNet()
model.load_state_dict(torch.load('model.pth'))
# 获取任意层的特征
def get_feature(image, layer_name):
model.eval()
features = []
def hook(module, input, output):
features.append(output.detach().numpy())
layer = model._modules.get(layer_name)
handle = layer.register_forward_hook(hook)
model(image)
handle.remove()
feature = np.concatenate(features, axis=0)
return feature
# 进行tsne可视化
def tsne_visualization(feature, label):
tsne = TSNE(n_components=2, init='pca', random_state=0)
feature_tsne = tsne.fit_transform(feature)
plt.scatter(feature_tsne[:,0], feature_tsne[:,1], c=label)
plt.show()
# 加载图片并进行tsne可视化
image = torch.randn(1, 3, 32, 32)
feature = get_feature(image, 'conv2')
label = [1]
tsne_visualization(feature, label)
```
你可以根据自己的需要,修改自己的网络模型和目标层名称,以及输入的图片和标签。
阅读全文
相关推荐
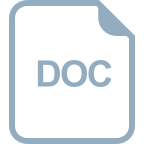
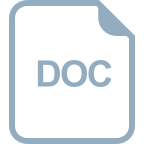
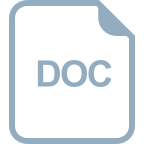
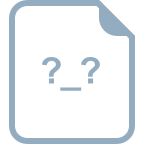
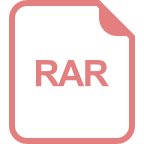
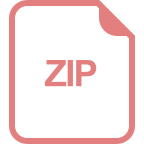
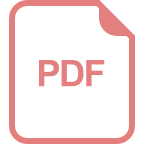
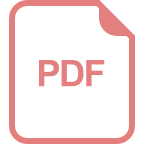
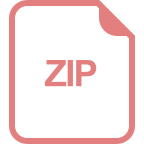
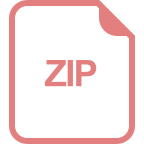
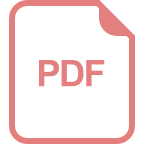
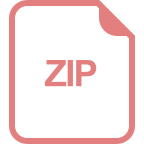
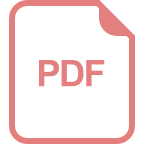
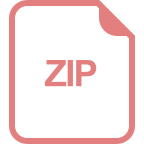
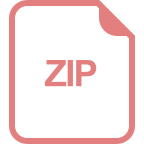
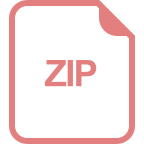
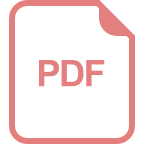
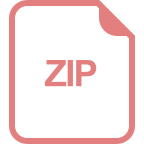