c语言二叉链表创建二叉树实现二叉树的基本功能完整代码
时间: 2024-12-12 12:14:10 浏览: 8
在C语言中,我们可以使用二叉链表来实现二叉树的数据结构。首先,我们需要定义一个二叉节点结构,包含值、左指针和右指针。然后,可以创建函数如插入、查找、删除等来实现基本的二叉树操作。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树节点结构
typedef struct TreeNode {
int val; // 节点值
struct TreeNode* left; // 左孩子指针
struct TreeNode* right; // 右孩子指针
} Node;
// 创建新节点
Node* createNode(int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (!newNode) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->val = value;
newNode->left = newNode->right = NULL;
return newNode;
}
// 插入节点到二叉树
Node* insertNode(Node* root, int value) {
if (root == NULL) {
return createNode(value);
}
if (value < root->val)
root->left = insertNode(root->left, value);
else if (value > root->val)
root->right = insertNode(root->right, value);
return root;
}
// 搜索节点
Node* searchNode(Node* root, int value) {
if (root == NULL || root->val == value)
return root;
if (value < root->val)
return searchNode(root->left, value);
else
return searchNode(root->right, value);
}
// 示例完整代码(这里仅提供核心部分,未包括其他辅助函数)
int main() {
Node* root = NULL; // 初始化根节点为空
// 插入一些元素(根据需要填充)
root = insertNode(root, 50);
insertNode(root, 30);
insertNode(root, 20);
insertNode(root, 40);
insertNode(root, 70);
insertNode(root, 60);
insertNode(root, 80);
// 查找示例
Node* found = searchNode(root, 60);
if (found != NULL)
printf("Found node with value %d\n", found->val);
else
printf("Node not found.\n");
return 0;
}
```
阅读全文
相关推荐
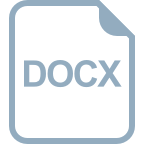
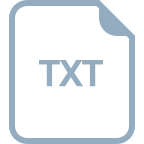
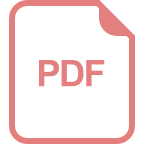





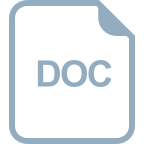









