以二叉链表作为二叉树的存储结构,编写c语言程序统计二叉树的叶结点个数。
时间: 2024-08-13 08:08:00 浏览: 83
在计算机科学中,二叉链表是一种常见的二叉树的存储结构,它将二叉树的节点组织成链表的形式。每个节点包含两个指向子节点的指针,通常是左孩子和右孩子,如果某个节点没有孩子,对应的指针就为NULL。
统计二叉树的叶结点(也称为终端节点或叶子节点)个数可以通过递归的方式实现,因为每个叶节点都没有子节点。下面是一个简单的C语言程序示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉链表节点结构
typedef struct Node {
int data;
struct Node* left;
struct Node* right;
} Node;
// 创建新节点
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode == NULL) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->data = data;
newNode->left = newNode->right = NULL;
return newNode;
}
// 判断是否为叶节点
int isLeaf(Node* node) {
return (node->left == NULL && node->right == NULL);
}
// 递归计算叶节点数量
int countLeaves(Node* root) {
if (root == NULL) {
return 0; // 如果根节点为空,叶节点个数为0
} else if (isLeaf(root)) {
return 1; // 如果当前节点是叶节点,返回1
} else {
// 递归计算左子树和右子树的叶节点个数,并加起来
return countLeaves(root->left) + countLeaves(root->right);
}
}
int main() {
// 假设这里已有一个构建好的二叉树的根节点
Node* root = ...; // 替换为实际的二叉树根节点
int leafCount = countLeaves(root);
printf("The number of leaf nodes in the binary tree is: %d\n", leafCount);
return 0;
}
```
在这个程序中,`countLeaves` 函数会遍历二叉树的每个节点,如果遇到叶节点,就递归地计数。最后在`main`函数中调用`countLeaves`并打印结果。
阅读全文
相关推荐
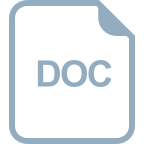
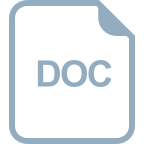
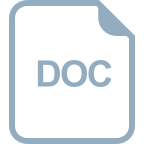















