编写脚本去处理这些序列:首先从每个序列中去掉引物部分,然后提取抗体序列。接着,找出这些抗体序列中哪些是重复的,并且确定它们之间的重复关系,最后输出结果。
时间: 2024-09-17 08:00:44 浏览: 36
编写这样一个脚本,通常会涉及到以下几个步骤:
1. **序列预处理**:首先,你需要读取包含引物和抗体序列的文件,可能是FASTA或CSV格式。使用适当的库(如Python的Biopython),你可以解析文件并提取出每个序列。通过查找特定的引物序列开始和结束位置(例如,基于已知引物长度),去掉引物部分。
```python
import Bio.SeqIO
def remove_primer(seq, primer_length):
if seq.startswith(primer_length * 'N') and seq.endswith('N' * primer_length): # 假设引物由固定数量的Ns组成
return seq[primer_length:-primer_length]
else:
return seq
sequences = []
for record in SeqIO.parse(input_file, "fasta"):
sequences.append(remove_primer(record.seq, primer_length))
```
2. **去重和关系确定**:使用集合数据结构(如Python的set)可以轻松地去除重复序列。对于序列关系的确定,你可能需要构建一个哈希表或者使用图算法(比如网络流或拓扑排序)。这里是一个简单的例子:
```python
unique_sequences = set(sequences)
duplicates = {seq: [seq] for seq in unique_sequences if sequences.count(seq) > 1}
# 简单的关系分析示例,只返回最频繁出现的重复序列
for seq, occurrences in duplicates.items():
most_frequent = max(occurrences, key=sequences.count)
print(f"{seq} is a duplicate of {most_frequent}, appears {occurrences.count(seq)} times")
```
3. **输出结果**:将去重后的唯一序列以及它们的重复信息保存到新的文件或者输出到控制台。
```python
with open(output_file, "w") as f:
for seq in sorted(unique_sequences):
f.write(f">{seq}\n{seq}\n")
print("\nDuplicate Sequences:")
for seq, duplicates in duplicates.items():
print(f"{seq}: {' '.join(duplicates)}")
```
阅读全文
相关推荐
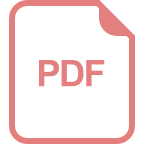
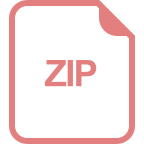
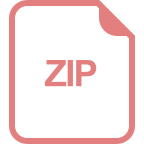
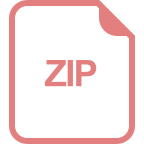
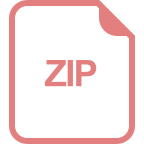
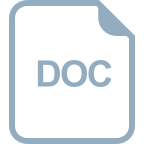
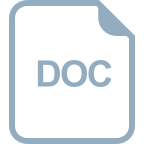
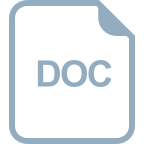
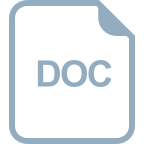
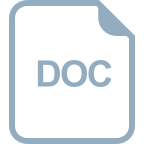
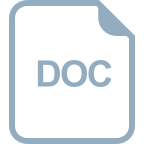
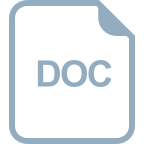
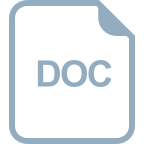
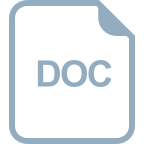
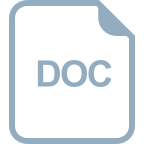

