python单链表的操作实现1)创建一个空的链表 (2)首端插入1,2,3 (3)尾端插入4,5,6,7,8,9,10 (4)首端弹出一个元素 (5)尾端弹出一个元素 (6)删除elem = 7的元素 (7)打印当前链表中的所有元素 (8)按下标查找并打印第3个结点的值。
时间: 2024-10-12 21:08:45 浏览: 38
在Python中,我们可以按照以下步骤实现单链表的操作:
1. **创建一个空的链表**:
```python
class Node:
def __init__(self, data=None):
self.data = data
self.next = None
head = Node() # 空链表的头节点
```
2. **首端插入1,2,3**:
```python
head = Node(1) # 插入第一个元素
current = head # 初始化当前节点
for i in [2, 3]:
new_node = Node(i)
new_node.next = current.next
current.next = new_node
```
3. **尾端插入4,5,6,7,8,9,10**:
```python
if not head.next: # 如果链表为空或只有一个元素
for num in range(4, 11):
head.next = Node(num)
head = head.next
else:
tail = head # 找到最后一个元素
for num in range(4, 11):
tail.next = Node(num)
tail = tail.next
```
4. **首端弹出一个元素**:
```python
if head is not None:
head = head.next # 首端移除当前节点
```
5. **尾端弹出一个元素**:
```python
if head and head.next: # 检查是否非空
tail = head
while tail.next.next: # 跳过最后一个元素
tail = tail.next
tail.next = None # 移除最后一个元素
```
6. **删除 elem = 7 的元素**:
```python
if head and head.data == 7:
head = head.next
else:
current = head
while current.next and current.next.data != 7:
current = current.next
if current.next:
current.next = current.next.next
```
7. **打印当前链表中的所有元素**:
```python
def print_list(head):
current = head
while current:
print(current.data, end=" -> ")
current = current.next
print("None")
print_list(head)
```
8. **按下标查找并打印第3个结点的值**:
由于Python的索引是从0开始的,所以第3个节点对应的是索引2。但是这里我们假设是按照链表顺序而非索引来获取值,可以稍作修改:
```python
def find_by_position(head, position=0):
count = 0
current = head
while current:
if count == position - 1:
print(current.data, "is the node at position", position)
break
current = current.next
count += 1
find_by_position(head, 3) # 查找第3个节点,注意位置从0开始
```
阅读全文
相关推荐
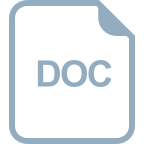
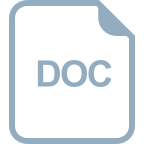
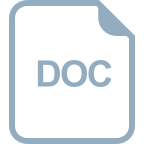
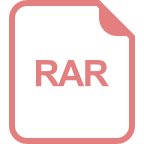
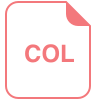













