串的BF算法,串的KMP算法c语言代码
时间: 2024-06-09 13:03:50 浏览: 148
BF(Boyer-Moore)算法和KMP(Knuth-Morris-Pratt)算法是两种常用的字符串匹配算法,它们在计算机科学中被广泛用于搜索文本中的模式串。
**BF算法**(Boyer-Moore算法),也称为坏字符法,它的核心思想是在匹配过程中跳过不匹配的字符,而不是回溯。算法主要包括两个部分:
1. **坏字符规则**:基于字符发生错误的情况,向右移动目标串,跳过的步长等于字符在模式串中出现的位置。
2. **好后缀规则**:如果找到一个字符与模式串的末尾匹配,会使用最长的相等子串作为跳过长度。
**KMP算法**(Knuth-Morris-Pratt算法),则是基于前缀函数的思想,通过预处理模式串创建一个部分匹配表,当遇到不匹配时,根据这个表跳过尽可能大的距离,避免回溯。
- **部分匹配表**(Pattern Matching Table, PMT):存储模式串的前缀和后缀公共部分的长度,用于快速定位。
以下是KMP算法的C语言代码示例:
```c
#include <stdio.h>
#include <string.h>
// 前缀函数计算函数
void compute_prefix_function(char* pattern, int prefix[], int n) {
prefix = 0;
int j = 0;
for (int i = 1; i < n; i++) {
while (j > 0 && pattern[i] != pattern[j]) {
j = prefix[j - 1];
}
if (pattern[i] == pattern[j]) {
j++;
}
prefix[i] = j;
}
}
// KMP搜索函数
int kmp_search(char* text, char* pattern) {
int m = strlen(pattern);
int n = strlen(text);
int i = 0, j = 0;
while (i < n && j < m) {
if (text[i] == pattern[j]) {
i++;
j++;
} else {
if (j != 0) {
j = prefix[j - 1];
} else {
i++;
}
}
}
if (j == m) return i - m; // 如果匹配成功,返回开始位置
return -1; // 匹配失败
}
int main() {
char text[] = "ABABDABACDABABCAB";
char pattern[] = "ABABCAB";
int prefix[m];
compute_prefix_function(pattern, prefix, m);
int result = kmp_search(text, pattern);
printf("Pattern found at position: %d\n", result);
return 0;
}
```
这两个算法各有优劣,BF算法对于不常见的字符错误处理较好,而KMP算法对于错误处理更高效,不需要回溯。如果你需要深入了解其中任意一种算法,可以提问相关的细节或者深入讲解。
阅读全文
相关推荐













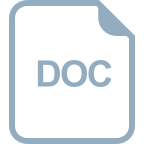
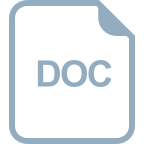