使用SQLAlchemy ORM(对象关系映射)来重写shmysql类以便于提供一个更为稳定和面向对象的方式与数据库交互
时间: 2024-10-10 16:11:59 浏览: 65
要使用SQLAlchemy ORM(对象关系映射)对`shmysql`类进行改写以实现更稳定的面向对象方式与数据库交互,首先需要安装并导入SQLAlchemy包,并定义对应的数据库模型。以下是一种可能的改写方式:
1. **安装SQLAlchemy**:如果尚未安装,请通过pip安装SQLAlchemy。
```bash
pip install sqlalchemy
```
2. **定义ORM模型**:创建代表数据库表的对象。
3. **建立数据库连接**:利用配置文件中的参数建立数据库会话。
4. **编写CRUD操作**:在新的类中实现增删查改的基本方法。
这里给出一个简化版的概念示例代码:
```python
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
import json
Base = declarative_base()
class MyTable(Base):
# 定义对应的数据表结构
__tablename__ = 'example_table'
id = Column(Integer, primary_key=True)
name = Column(String(50))
value = Column(String(50))
class SQLAlchemyWrapper:
def __init__(self):
with open('./shmysql.json', 'r', encoding='utf8') as fp:
config = json.load(fp)
engine = create_engine(f"mysql+pymysql://{config['user']}:{config['password']}@{config['host']}/{config['database']}")
Base.metadata.create_all(engine)
Session = sessionmaker(bind=engine)
self.session = Session()
def add_record(self, record):
""" 添加记录 """
self.session.add(record)
self.session.commit()
def query_records(self, model_class, **kwargs):
""" 查询记录 """
return self.session.query(model_class).filter_by(**kwargs).all()
def update_record(self, instance, **updates):
""" 更新记录 """
for key, value in updates.items():
setattr(instance, key, value)
self.session.commit()
def delete_record(self, instance):
""" 删除记录 """
self.session.delete(instance)
self.session.commit()
def close(self):
""" 关闭session """
self.session.close()
# 使用例子
if __name__ == "__main__":
db = SQLAlchemyWrapper()
new_record = MyTable(name='Example', value='Value')
db.add_record(new_record)
records = db.query_records(MyTable, name='Example')
print(records[0].value)
```
这段代码展示了如何用SQLAlchemy ORM重写`shmysql`类,包括了基本的数据库操作如添加、查询、更新和删除数据等。请注意实际应用时还需根据具体的业务逻辑调整模型定义及操作方法。
阅读全文
相关推荐
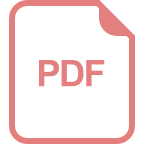
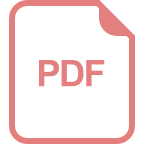
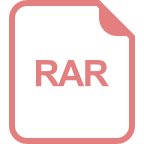

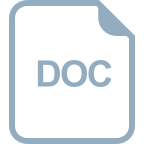
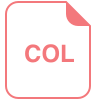
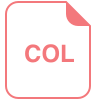
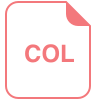
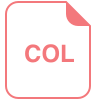
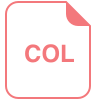
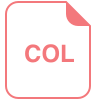
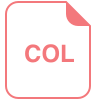
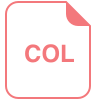
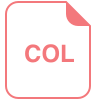
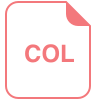
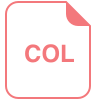
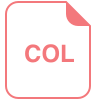
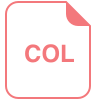
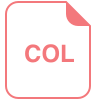