SSTF算法用c语言实现
时间: 2025-01-01 10:33:55 浏览: 9
SSTF(Shortest Job First, 短作业优先)算法是一个非抢占式的作业调度算法,它假设每个进程都有一个确定的运行时间。在C语言中实现SSTF算法,我们通常会创建一个结构体来表示进程,包含进程ID、运行时间和一个标志来记录是否正在执行。以下是一个简单的步骤描述:
1. 定义一个进程结构体(Process),包含进程ID(pid)、运行时间(execution_time)和一个标志(is_running):
```c
typedef struct {
int pid;
int execution_time;
bool is_running; // 标志位
} Process;
```
2. 创建一个空的任务列表(例如使用链表):
```c
struct List {
Process *head;
Process *tail;
};
List job_queue = {NULL, NULL};
```
3. 实现插入新任务的功能,并保持SSTF顺序:
```c
void insertTask(int pid, int exec_time) {
Process new_task = {pid, exec_time, false};
if (job_queue.head == NULL) {
job_queue.head = job_queue.tail = &new_task;
} else {
if (new_task.execution_time < job_queue.tail->execution_time) {
new_task.is_running = true;
job_queue.tail->is_running = false;
job_queue.tail = &new_task;
} else {
Process* temp = job_queue.head;
while (temp->next != NULL && temp->next->execution_time < new_task.execution_time) {
temp = temp->next;
}
new_task.is_running = true;
temp->next = &new_task;
if (temp == job_queue.head) {
job_queue.head = new_task;
}
}
}
}
```
4. 模拟调度过程,选择并执行最短的任务:
```c
void schedule() {
while (!job_queue.head->is_running) {
if (job_queue.head != NULL) {
job_queue.head->is_running = true;
printf("Executing task %d with time %d\n", job_queue.head->pid, job_queue.head->execution_time);
// 这里模拟任务执行,完成后更新状态...
job_queue.head->execution_time -= 1; // 假设单位时间内执行完一个任务
if (job_queue.head->execution_time <= 0) {
job_queue.head->is_running = false;
job_queue.head = job_queue.head->next;
if (job_queue.head == NULL) {
job_queue.tail = NULL;
}
}
} else {
break; // 所有任务已完成
}
}
}
```
这只是一个简化的示例,实际应用中还需要考虑更多的边缘情况和错误处理。
阅读全文
相关推荐














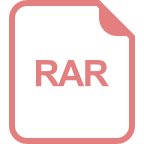
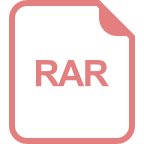

