磁盘调度算法c语言实现
时间: 2023-10-08 11:13:37 浏览: 115
以下是几种常见磁盘调度算法的C语言实现:
1. 先来先服务(FCFS)算法
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
int main()
{
int queue[1000], head, n, i, j, seek_time = 0;
printf("Enter the number of requests: ");
scanf("%d", &n);
printf("Enter the request queue: ");
for(i=0; i<n; i++)
scanf("%d", &queue[i]);
printf("Enter the initial head position: ");
scanf("%d", &head);
for(i=0; i<n; i++)
{
seek_time += abs(head - queue[i]);
head = queue[i];
}
printf("Total seek time: %d\n", seek_time);
return 0;
}
```
2. 最短寻道时间优先(SSTF)算法
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
int find_closest(int queue[], int n, int head, int visited[])
{
int min_dist = 100000, index = -1, i;
for(i=0; i<n; i++)
{
if(!visited[i])
{
int dist = abs(head - queue[i]);
if(dist < min_dist)
{
index = i;
min_dist = dist;
}
}
}
return index;
}
int main()
{
int queue[1000], head, n, i, j, seek_time = 0, visited[1000] = {0};
printf("Enter the number of requests: ");
scanf("%d", &n);
printf("Enter the request queue: ");
for(i=0; i<n; i++)
scanf("%d", &queue[i]);
printf("Enter the initial head position: ");
scanf("%d", &head);
for(i=0; i<n; i++)
{
int index = find_closest(queue, n, head, visited);
visited[index] = 1;
seek_time += abs(head - queue[index]);
head = queue[index];
}
printf("Total seek time: %d\n", seek_time);
return 0;
}
```
3. 扫描(SCAN)算法
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
int main()
{
int queue[1000], head, n, i, j, seek_time = 0;
printf("Enter the number of requests: ");
scanf("%d", &n);
printf("Enter the request queue: ");
for(i=0; i<n; i++)
scanf("%d", &queue[i]);
printf("Enter the initial head position: ");
scanf("%d", &head);
int direction = 1; // 1 for up, -1 for down
for(i=0; i<n; i++)
{
if(direction == 1) // scanning up
{
int closest_index = -1, min_dist = 100000;
for(j=0; j<n; j++)
{
if(queue[j] >= head && abs(queue[j] - head) < min_dist)
{
closest_index = j;
min_dist = abs(queue[j] - head);
}
}
if(closest_index == -1) // no requests above head
{
direction = -1;
seek_time += abs(queue[n-1] - head); // go to the end
head = queue[n-1];
}
else
{
seek_time += abs(queue[closest_index] - head);
head = queue[closest_index];
}
}
else // scanning down
{
int closest_index = -1, min_dist = 100000;
for(j=0; j<n; j++)
{
if(queue[j] <= head && abs(queue[j] - head) < min_dist)
{
closest_index = j;
min_dist = abs(queue[j] - head);
}
}
if(closest_index == -1) // no requests below head
{
direction = 1;
seek_time += abs(queue[0] - head); // go to the beginning
head = queue[0];
}
else
{
seek_time += abs(queue[closest_index] - head);
head = queue[closest_index];
}
}
}
printf("Total seek time: %d\n", seek_time);
return 0;
}
```
4. 循环扫描(C-SCAN)算法
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
int main()
{
int queue[1000], head, n, i, j, seek_time = 0;
printf("Enter the number of requests: ");
scanf("%d", &n);
printf("Enter the request queue: ");
for(i=0; i<n; i++)
scanf("%d", &queue[i]);
printf("Enter the initial head position: ");
scanf("%d", &head);
int direction = 1; // 1 for up, -1 for down
for(i=0; i<n; i++)
{
if(direction == 1) // scanning up
{
int closest_index = -1, min_dist = 100000;
for(j=0; j<n; j++)
{
if(queue[j] >= head && abs(queue[j] - head) < min_dist)
{
closest_index = j;
min_dist = abs(queue[j] - head);
}
}
if(closest_index == -1) // no requests above head
{
direction = -1;
seek_time += abs(queue[n-1] - head) + abs(queue[n-1] - queue[0]); // go to the end and then to the beginning
head = queue[0];
}
else
{
seek_time += abs(queue[closest_index] - head);
head = queue[closest_index];
}
}
else // scanning down
{
int closest_index = -1, min_dist = 100000;
for(j=0; j<n; j++)
{
if(queue[j] <= head && abs(queue[j] - head) < min_dist)
{
closest_index = j;
min_dist = abs(queue[j] - head);
}
}
if(closest_index == -1) // no requests below head
{
direction = 1;
seek_time += abs(queue[n-1] - head) + abs(queue[n-1] - queue[0]); // go to the end and then to the beginning
head = queue[0];
}
else
{
seek_time += abs(queue[closest_index] - head);
head = queue[closest_index];
}
}
}
printf("Total seek time: %d\n", seek_time);
return 0;
}
```
阅读全文
相关推荐
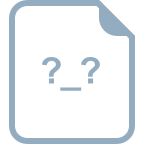
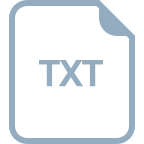












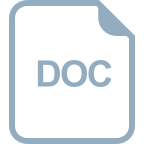
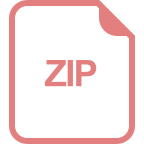
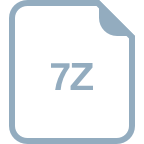