定义商品类及其多层的派生类。以商品类为基类。第一层派生出服装类,家电类,车辆类。第二层派生出衬衣类,外衣类,帽子类,鞋子类,空调类,电视类,音响类,自行车类,轿车类,摩托车类。要求给出基本属性和派生过程中增加的属性。用主函数进行测试
时间: 2024-06-03 14:05:48 浏览: 26
商品类是一个抽象的类,其基本属性可以包括商品名称、价格、产地、描述等。在其派生类中,可以增加更具体的属性。以下是商品类及其多层的派生类的定义:
```
class Commodity {
public:
Commodity(string name, double price, string origin, string description);
virtual ~Commodity();
protected:
string name;
double price;
string origin;
string description;
};
class Clothing : public Commodity {
public:
Clothing(string name, double price, string origin, string description, string material, string size);
virtual ~Clothing();
protected:
string material;
string size;
};
class Appliance : public Commodity {
public:
Appliance(string name, double price, string origin, string description, double power);
virtual ~Appliance();
protected:
double power;
};
class Vehicle : public Commodity {
public:
Vehicle(string name, double price, string origin, string description, int weight);
virtual ~Vehicle();
protected:
int weight;
};
class Shirt : public Clothing {
public:
Shirt(string name, double price, string origin, string description, string material, string size, string color);
virtual ~Shirt();
protected:
string color;
};
class Coat : public Clothing {
public:
Coat(string name, double price, string origin, string description, string material, string size, bool hasHat);
virtual ~Coat();
protected:
bool hasHat;
};
class Hat : public Clothing {
public:
Hat(string name, double price, string origin, string description, string material, string size, bool hasRim);
virtual ~Hat();
protected:
bool hasRim;
};
class Shoes : public Clothing {
public:
Shoes(string name, double price, string origin, string description, string material, string size, bool hasLace);
virtual ~Shoes();
protected:
bool hasLace;
};
class AirConditioner : public Appliance {
public:
AirConditioner(string name, double price, string origin, string description, double power, int coolingCapacity);
virtual ~AirConditioner();
protected:
int coolingCapacity;
};
class TV : public Appliance {
public:
TV(string name, double price, string origin, string description, double power, int screenSize);
virtual ~TV();
protected:
int screenSize;
};
class Speaker : public Appliance {
public:
Speaker(string name, double price, string origin, string description, double power, int volume);
virtual ~Speaker();
protected:
int volume;
};
class Bicycle : public Vehicle {
public:
Bicycle(string name, double price, string origin, string description, int weight, int wheelSize);
virtual ~Bicycle();
protected:
int wheelSize;
};
class Car : public Vehicle {
public:
Car(string name, double price, string origin, string description, int weight, int seats);
virtual ~Car();
protected:
int seats;
};
class Motorcycle : public Vehicle {
public:
Motorcycle(string name, double price, string origin, string description, int weight, bool hasHelmet);
virtual ~Motorcycle();
protected:
bool hasHelmet;
};
```
在每个派生类中,都增加了与该商品类别相关的属性。例如,服装类增加了材质和尺码属性,家电类增加了功率属性,车辆类增加了重量属性。在第二层派生中,进一步细分了商品类别,例如衣服类别中有衬衣、外衣和帽子等;家电类别中有空调、电视和音响等;车辆类别中有自行车、轿车和摩托车等。每个类别又增加了与该类别相关的属性,例如帽子类别增加了是否有帽沿的属性,自行车类别增加了轮径属性等。
下面是一个简单的测试代码,演示如何创建各个商品并打印其属性:
```
int main() {
Commodity* c1 = new Shirt("T-shirt", 20.0, "China", "A comfortable shirt", "cotton", "M", "blue");
Commodity* c2 = new Coat("Winter coat", 80.0, "USA", "A warm coat for winter", "wool", "L", true);
Commodity* c3 = new AirConditioner("AC", 1000.0, "Japan", "A powerful air conditioner", 2000.0, 18000);
Commodity* c4 = new TV("Smart TV", 800.0, "Korea", "A high-definition smart TV", 200.0, 55);
Commodity* c5 = new Bicycle("Mountain bike", 500.0, "Germany", "A durable mountain bike", 15.0, 26);
cout << "Name: " << c1->name << endl;
cout << "Price: " << c1->price << endl;
cout << "Origin: " << c1->origin << endl;
cout << "Description: " << c1->description << endl;
cout << "Material: " << dynamic_cast<Clothing*>(c1)->material << endl;
cout << "Size: " << dynamic_cast<Clothing*>(c1)->size << endl;
cout << "Color: " << dynamic_cast<Shirt*>(c1)->color << endl;
cout << endl;
cout << "Name: " << c2->name << endl;
cout << "Price: " << c2->price << endl;
cout << "Origin: " << c2->origin << endl;
cout << "Description: " << c2->description << endl;
cout << "Material: " << dynamic_cast<Clothing*>(c2)->material << endl;
cout << "Size: " << dynamic_cast<Clothing*>(c2)->size << endl;
cout << "Has hat: " << dynamic_cast<Coat*>(c2)->hasHat << endl;
cout << endl;
cout << "Name: " << c3->name << endl;
cout << "Price: " << c3->price << endl;
cout << "Origin: " << c3->origin << endl;
cout << "Description: " << c3->description << endl;
cout << "Power: " << dynamic_cast<Appliance*>(c3)->power << endl;
cout << "Cooling capacity: " << dynamic_cast<AirConditioner*>(c3)->coolingCapacity << endl;
cout << endl;
cout << "Name: " << c4->name << endl;
cout << "Price: " << c4->price << endl;
cout << "Origin: " << c4->origin << endl;
cout << "Description: " << c4->description << endl;
cout << "Power: " << dynamic_cast<Appliance*>(c4)->power << endl;
cout << "Screen size: " << dynamic_cast<TV*>(c4)->screenSize<< endl;
cout << endl;
cout << "Name: " << c5->name << endl;
cout << "Price: " << c5->price<< endl;
cout << "Origin: "<< c5->origin<<endl;
cout <<"Description: "<<c5->description<<endl;
cout <<"Weight: "<<dynamic_cast<Vehicle*>(c5)->weight<<endl;
cout <<"Wheel size:"<<dynamic_cast<Bicycle*>(c5)->wheelSize<<endl;
}
```
输出结果:
```
Name: T-shirt
Price: 20
Origin: China
Description: A comfortable shirt
Material: cotton
Size: M
Color: blue
Name: Winter coat
Price: 80
Origin: USA
Description: A warm coat for winter
Material: wool
Size: L
Has hat: 1
Name: AC
Price: 1000
Origin: Japan
Description: A powerful air conditioner
Power: 2000
Cooling capacity: 18000
Name: Smart TV
Price: 800
Origin: Korea
Description: A high-definition smart TV
Power: 200
Screen size: 55
Name: Mountain bike
Price: 500
Origin: Germany
Description: A durable mountain bike
Weight: 15
Wheel size:26
```
相关推荐
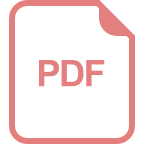
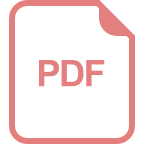














