使用【c++】写【文本相似度分析】的代码
时间: 2024-10-08 11:27:20 浏览: 48
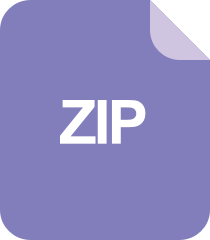
c++程序实现c++代码相似度计算

使用 C++ 编写文本相似度分析的代码可以采用一些常见的算法,比如余弦相似度算法。具体实现过程可以分为以下几个步骤:
1. 对文本进行预处理,比如去除停用词、标点符号等。
2. 将每个文本转换为向量表示,可以使用词袋模型或 TF-IDF 模型进行向量化。
3. 对两个文本的向量进行余弦相似度计算,得出相似度分数。
4. 可以设置阈值来判断两个文本是否相似。
下面是一个简单的 C++ 代码示例,用于计算两个文本之间的余弦相似度:
```c++
#include <iostream>
#include <string>
#include <vector>
#include <unordered_map>
#include <cmath>
using namespace std;
// 计算两个向量之间的余弦相似度
double cosine_similarity(const vector<double>& v1, const vector<double>& v2) {
double dot_product = 0.0;
double norm_v1 = 0.0;
double norm_v2 = 0.0;
for (int i = 0; i < v1.size(); i++) {
dot_product += v1[i] * v2[i];
norm_v1 += pow(v1[i], 2);
norm_v2 += pow(v2[i], 2);
}
norm_v1 = sqrt(norm_v1);
norm_v2 = sqrt(norm_v2);
return dot_product / (norm_v1 * norm_v2);
}
// 对文本进行预处理,去除停用词和标点符号
string preprocess_text(const string& text) {
unordered_map<string, bool> stop_words = {
{"the", true},
{"and", true},
{"a", true},
{"an", true},
{"in", true},
{"on", true},
{"at", true},
{"to", true},
{"for", true},
{"with", true}
};
string result;
for (char c : text) {
if (isalpha(c)) {
result += tolower(c);
} else if (isspace(c)) {
result += ' ';
}
}
string word;
for (int i = 0; i < result.length(); i++) {
if (result[i] == ' ') {
if (!word.empty() && !stop_words[word]) {
word += ' ';
}
word.clear();
} else {
word += result[i];
}
}
if (!word.empty() && !stop_words[word]) {
result += word;
}
return result;
}
// 将文本转换为向量表示,使用词袋模型
vector<double> bag_of_words(const string& text, const unordered_map<string, int>& vocab) {
vector<double> result(vocab.size(), 0.0);
string word;
for (int i = 0; i < text.length(); i++) {
if (text[i] == ' ' || i == text.length() - 1) {
if (!word.empty()) {
if (vocab.count(word)) {
result[vocab.at(word)]++;
}
word.clear();
}
} else {
word += text[i];
}
}
return result;
}
int main() {
// 定义两个文本
string text1 = "I love programming";
string text2 = "Programming is my passion";
// 预处理文本
text1 = preprocess_text(text1);
text2 = preprocess_text(text2);
// 建立词汇表
unordered_map<string, int> vocab;
string word;
for (char c : text1 + text2) {
if (c == ' ' && !word.empty()) {
if (!vocab.count(word)) {
vocab.emplace(word, vocab.size());
}
word.clear();
} else if (isalpha(c)) {
word += tolower(c);
}
}
// 将文本转换为向量表示
auto vec1 = bag_of_words(text1, vocab);
auto vec2 = bag_of_words(text2, vocab);
// 计算余弦相似度
double similarity_score = cosine_similarity(vec1, vec2);
cout << "The similarity score is: " << similarity_score << endl;
return 0;
}
```
阅读全文
相关推荐
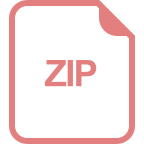
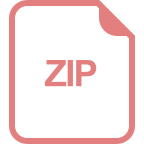




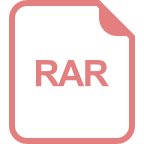
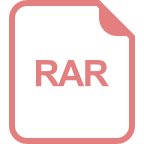
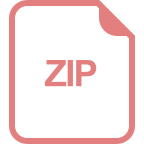
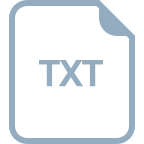
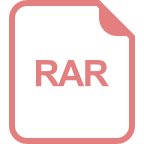
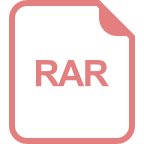
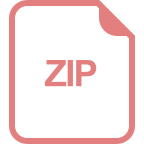
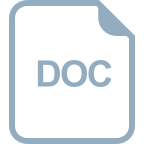
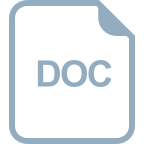


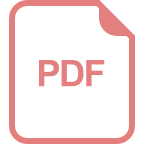