使用java对矩阵类matrix进行进化抽象,让其支持通过读取文本文件中的数据来创建或初始化矩阵数据,并提供矩阵的加减乘除运算,如果两个矩阵不符合矩阵的运算规则时,请抛出异常对象
时间: 2024-12-12 09:01:16 浏览: 9
在Java中,我们可以设计一个Matrix类来进行矩阵操作的抽象,使其具备从文本文件加载数据的功能。首先,我们需要实现以下关键特性:
1. **抽象类或接口**:
```java
// Matrix抽象类
public abstract class Matrix<T extends Number> {
private int rows;
private int cols;
private T[][] data;
public Matrix(int rows, int cols) {
this.rows = rows;
this.cols = cols;
data = new T[rows][cols];
}
// 其他基本方法如获取行列数、大小等
// 抽象方法,用于从文件加载数据
public abstract void loadFromTextFile(String filePath);
// 矩阵运算方法,抛出异常
public T[][] add(T[][] other) throws MatrixException {
checkCompatibility(other);
// 实现矩阵相加
}
public T[][] subtract(T[][] other) throws MatrixException {
checkCompatibility(other);
// 实现矩阵相减
}
public T[][] multiply(T[][] other) throws MatrixException {
checkCompatibility(other);
// 实现矩阵相乘
}
public T[][] divide(T[][] other) throws MatrixException {
checkCompatibility(other);
// 实现矩阵相除
}
private void checkCompatibility(T[][] other) throws MatrixException {
if (other == null || other.length != rows || other[0].length != cols) {
throw new MatrixException("Incompatible matrix size");
}
}
}
// 特定类型的矩阵实现,如DoubleMatrix或IntegerMatrix
class DoubleMatrix extends Matrix<Double> {
// 实现loadFromTextFile方法,解析double数组
}
```
2. **自定义异常类**:
```java
class MatrixException extends Exception {
public MatrixException(String message) {
super(message);
}
}
```
阅读全文
相关推荐







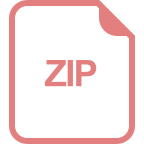









