Error in v-on handler: "ReferenceError: dialogFormVisible is not defined"
时间: 2024-04-22 11:24:12 浏览: 28
这个错误通常是因为在Vue组件中使用了一个未定义的变量dialogFormVisible。要解决这个错误,你可以按照以下步骤进行检查和修复:
1. 确保在Vue组件的data属性中定义了dialogFormVisible变量,并且它被正确初始化。例如:
```
data() {
return {
dialogFormVisible: false
}
}
```
2. 检查你的模板代码,确保在使用v-on指令时正确引用了dialogFormVisible变量。例如:
```
<button v-on:click="dialogFormVisible = !dialogFormVisible">Toggle Dialog</button>
```
3. 如果你在组件中的方法中使用了dialogFormVisible变量,确保在方法内部也定义了该变量。例如:
```
methods: {
showDialog() {
this.dialogFormVisible = true;
}
}
```
通过检查和修复以上步骤,你应该能够解决这个错误。如果问题仍然存在,可以提供更多的代码和错误信息,以便我能够给出更具体的建议。
相关问题
Error in v-on handler: "ReferenceError: isCollect is not defined"
根据引用\[1\]中的解释,问题出现在v-on命令中的isCollect变量没有定义。你在代码中已经在data中定义了isCollect变量,但是在方法中使用实例上的变量时,必须使用this来指向这个变量。所以,你需要在v-on命令中使用this.isCollect来引用isCollect变量,这样问题就可以解决了。
#### 引用[.reference_title]
- *1* [Error in v-on handler: “ReferenceError: isShow is not defined](https://blog.csdn.net/m0_50013284/article/details/125717671)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* [Error in v-on handler: “ReferenceError: state is not defined](https://blog.csdn.net/linduj/article/details/127211351)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *3* [Vue.js报错问题解决:[Vue warn]: Error in v-on handler: “ReferenceError: XXX is not defined“.](https://blog.csdn.net/m0_56426418/article/details/130162657)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
Error in v-on handler: "ReferenceError: id is not defined"
This error message usually occurs when you are trying to access a variable or property that has not been defined or initialized. In this case, it seems that the variable "id" is not defined in the context where the v-on handler is being used.
To fix this error, you need to make sure that the "id" variable is declared and initialized before it is used in the v-on handler. You can do this by defining the "id" variable in the component's data object or by passing it as a prop from a parent component.
For example, if you are using the v-on directive to handle a click event on a button and you want to pass the "id" of the clicked button to a method, you can define the "id" variable in the component's data object like this:
```
<template>
<button v-for="item in items" v-bind:key="item.id" v-on:click="handleClick(item.id)">
{{ item.name }}
</button>
</template>
<script>
export default {
data() {
return {
items: [
{ id: 1, name: 'Item 1' },
{ id: 2, name: 'Item 2' },
{ id: 3, name: 'Item 3' }
]
}
},
methods: {
handleClick(id) {
console.log('Clicked item with id:', id)
}
}
}
</script>
```
In this example, the "id" variable is passed as an argument to the "handleClick" method when the button is clicked. The "handleClick" method then logs the "id" to the console.
相关推荐
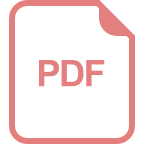
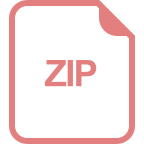
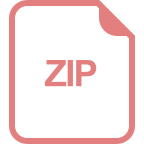












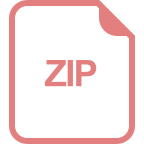