用C语言分别编写两个程序,实现fread和fwrite函数
时间: 2024-11-06 19:18:58 浏览: 25
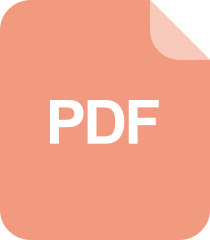
C语言fread函数和fwrite函数的用法及其实例

在C语言中,`fread` 和 `fwrite` 是标准库函数,它们用于文件操作,分别用于从文件读取数据和向文件写入数据。由于这两个函数的具体实现涉及到操作系统底层细节,我们不会在这里直接提供完整的源代码,但我会给你大致的函数原型和简述如何使用。
**fread 函数原型:**
```c
size_t fread(void *ptr, size_t size, size_t count, FILE *stream);
```
这个函数接受四个参数:
- `ptr`: 要存储读取数据的内存地址。
- `size`: 单个元素的大小(字节)。
- `count`: 要读取的元素数目。
- `stream`: 指向要读取的文件的指针,通常由 `fopen` 返回。
**fwrite 函数原型:**
```c
size_t fwrite(const void *ptr, size_t size, size_t count, FILE *stream);
```
这里的参数含义与 `fread` 相同,区别在于 `const void *ptr` 表示你要写入的数据是常量指向的。
以下是使用这两个函数的基本示例,假设你想读取一个整数数组并写入到另一个文件:
```c
#include <stdio.h>
// 读取文件内容到数组
void read_file(int *array, size_t n, FILE *input) {
size_t bytes_read = fread(array, sizeof(int), n, input);
if (bytes_read != n) {
perror("Error reading from file");
}
}
// 将数组内容写入到文件
void write_file(const int *array, size_t n, FILE *output) {
size_t bytes_written = fwrite(array, sizeof(int), n, output);
if (bytes_written != n) {
perror("Error writing to file");
}
}
int main() {
// 打开输入文件和输出文件
FILE *input = fopen("input.txt", "r");
FILE *output = fopen("output.txt", "w");
if (input == NULL || output == NULL) {
perror("Error opening files");
return 1;
}
// 读取数据
int numbers[n];
read_file(numbers, n, input);
// 写入数据
write_file(numbers, n, output);
fclose(input);
fclose(output);
return 0;
}
```
阅读全文
相关推荐
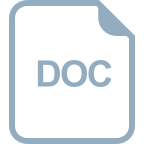
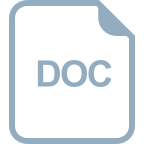
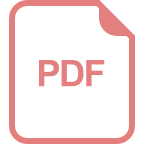
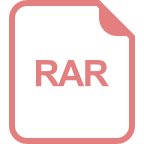


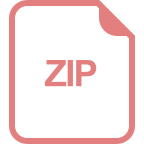
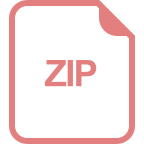
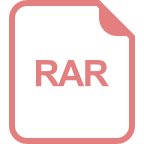
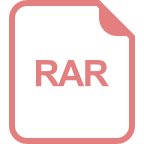
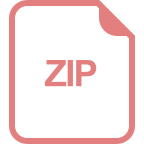
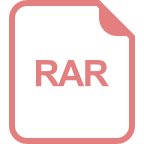
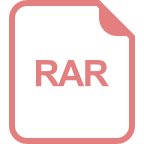
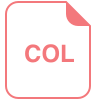

