javafx读取Outlook邮件
时间: 2023-08-11 18:04:46 浏览: 141
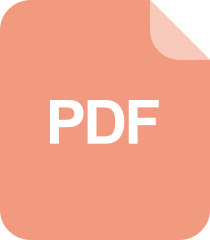
Pro JavaFX 2.pdf
你可以使用JavaMail API来读取Outlook邮件。以下是一个使用JavaFX和JavaMail API的示例代码,用于连接到Outlook邮件服务器并读取邮件:
```java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.TextArea;
import javafx.stage.Stage;
import java.util.Properties;
import javax.mail.*;
public class OutlookMailReader extends Application {
private String username = "your_email@example.com";
private String password = "your_password";
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
TextArea textArea = new TextArea();
textArea.setEditable(false);
Properties props = new Properties();
props.setProperty("mail.store.protocol", "imaps");
try {
Session session = Session.getInstance(props, null);
Store store = session.getStore();
store.connect("outlook.office365.com", username, password);
Folder inbox = store.getFolder("INBOX");
inbox.open(Folder.READ_ONLY);
Message[] messages = inbox.getMessages();
for (Message message : messages) {
textArea.appendText("From: " + message.getFrom()[0] + "\n");
textArea.appendText("Subject: " + message.getSubject() + "\n");
textArea.appendText("Content: " + message.getContent().toString() + "\n");
textArea.appendText("-------------------------------------------\n");
}
inbox.close(false);
store.close();
} catch (Exception e) {
e.printStackTrace();
}
primaryStage.setScene(new Scene(textArea, 600, 400));
primaryStage.show();
}
}
```
请注意,你需要将`your_email@example.com`和`your_password`替换为你的Outlook邮件账户的实际凭据。此外,你需要确保在项目的构建路径中包含JavaMail库和相关的依赖。
该示例代码创建了一个带有文本区域的JavaFX应用程序窗口。它使用JavaMail API来连接到Outlook邮件服务器,并从收件箱中获取邮件。然后,它将发件人,主题和邮件内容显示在文本区域中。
请注意,为了安全起见,你应该将敏感信息(如用户名和密码)保存在安全的位置,而不是直接在代码中硬编码。
希望这可以帮助到你!如果有任何进一步的问题,请随时提问。
阅读全文
相关推荐
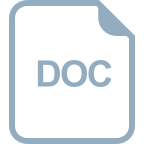
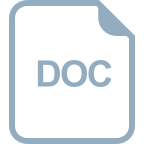
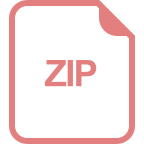
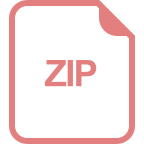
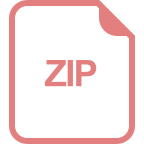
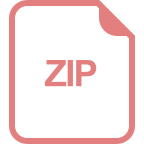
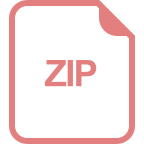
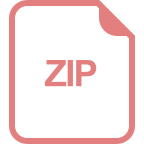
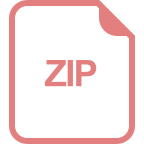
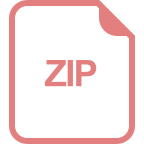
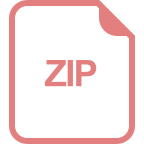
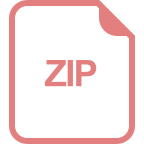
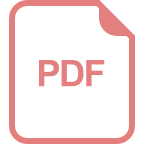