vue3点击父组件想调用子组件的函数应该怎么写
时间: 2024-12-09 13:13:34 浏览: 6
在Vue 3中,如果你想在父组件中点击某个元素时调用子组件的方法,可以通过以下几种方式实现:
1. **使用ref引用子组件**:
- 在父组件中为子组件添加`ref`属性。
- 在父组件的方法中通过`this.$refs`引用子组件并调用其方法。
2. **使用事件总线**:
- 创建一个事件总线(EventBus),在子组件中监听事件,在父组件中触发事件。
3. **使用provide/inject**:
- 在父组件中使用`provide`提供方法,在子组件中使用`inject`注入并调用该方法。
以下是使用`ref`引用的示例:
**父组件(ParentComponent.vue)**:
```vue
<template>
<div>
<button @click="callChildMethod">点击调用子组件方法</button>
<ChildComponent ref="childRef" />
</div>
</template>
<script>
import { ref } from 'vue';
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
setup() {
const childRef = ref(null);
const callChildMethod = () => {
if (childRef.value) {
childRef.value.childMethod();
}
};
return {
childRef,
callChildMethod
};
}
};
</script>
```
**子组件(ChildComponent.vue)**:
```vue
<template>
<div>
<p>这是子组件</p>
</div>
</template>
<script>
export default {
methods: {
childMethod() {
console.log('子组件的方法被调用了');
}
}
};
</script>
```
在这个示例中,当父组件的按钮被点击时,`callChildMethod`方法会被调用,该方法通过`childRef`引用子组件并调用其`childMethod`方法。
阅读全文
相关推荐
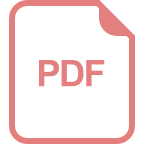
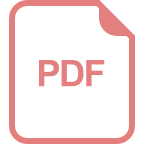
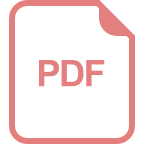
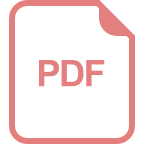
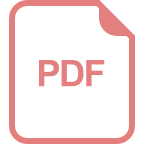
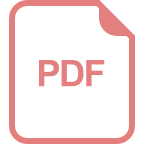
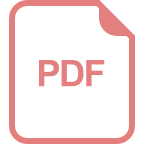
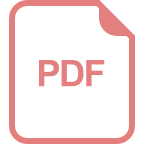
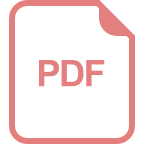
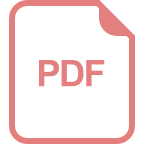
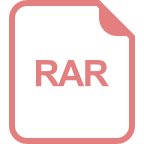
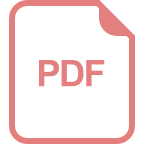
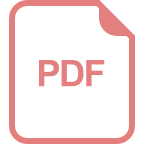
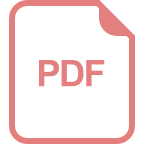

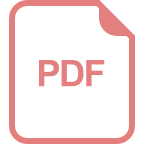