如何定义并实现一个函数来判断和规范化给定字符串s?该过程涉及到检查是否存在特定子串t,如果存在则删除第一个出现的t,并在字符串末尾追加字符串p,重复此过程直到字符串s满足规范条件。能否详细说明这个算法的步骤以及相应C语言的编程实现?
时间: 2024-10-21 21:02:34 浏览: 45
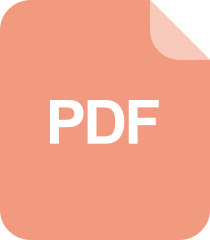
使用C语言提取子字符串及判断对称子字符串最大长度
为了定义并实现一个这样的函数,我们可以按照以下步骤进行:
1. 定义一个名为`normalize_string`的函数,接受两个输入参数:`s`(待处理的字符串),`t`(需要查找和移除的子串),以及`p`(添加到字符串末尾的字符串)。
2. 使用循环结构(如`while`循环),检查`s`是否包含`t`。
3. 如果找到`t`,使用`str_remove_first_occurrence`或`strstr`函数(C标准库中的函数,或者自定义一个函数)删除`s`的第一个出现的`t`。
4. 更新`s`为删除`t"之后的结果。
5. 检查`s`是否仍然包含`t`,如果不包含,跳出循环;否则继续迭代。
6. 当`s`不再需要修改(即不含`t`)时,将`p`追加到`s`的末尾。
7. 函数返回经过规范化处理后的字符串`s`。
下面是C语言的一个简单示例实现:
```c
#include <stdio.h>
#include <string.h>
// 自定义函数,用于删除字符串中第一个出现的子串
void remove_first_occurrence(char* str, char* substr) {
char* pos = strstr(str, substr);
if (pos) {
memmove(pos, pos + strlen(substr), strlen(str) - pos + 1);
}
}
char* normalize_string(const char* s, const char* t, const char* p) {
while (strstr(s, t)) {
remove_first_occurrence(s, t);
}
// 添加p到字符串末尾
strcat(s, p);
return s;
}
int main() {
char input[] = "Hello, there! How are you? I am fine.";
char to_remove[] = "you";
char add[] = ", nice to meet.";
char* normalized = normalize_string(input, to_remove, add);
printf("Normalized string: %s\n", normalized);
return 0;
}
```
在这个例子中,`normalize_string`会返回一个新字符串:"Hello, there! How is I am fine., nice to meet."
阅读全文
相关推荐
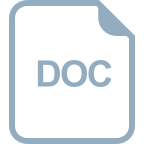
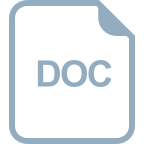
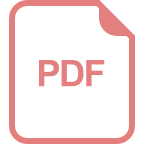














