针对带表头结点的单链表,试编写下列函数。 (1) 定位函数Locate:在单链表中寻找第i个结点。若找到,则函数返回第i个结点的地址;若找不到,则函数返回NULL。 (2) 求最大值函数max:通过一趟遍历在单链表中确定值最大的结点。
时间: 2024-09-30 21:13:22 浏览: 65
针对带表头结点的单链表,我们可以分别编写如下的函数:
(1) 定位函数 Locate (在链表中定位第 i 个节点)
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
Node* Locate(Node* head, int i) {
if (head == NULL || i <= 0) {
return NULL; // 列表为空或索引越界,返回 NULL
}
Node* current = head;
for (int j = 1; j < i && current != NULL; j++) {
current = current->next;
}
if (current != NULL) {
return current; // 找到了第 i 个节点,返回其指针
} else {
return NULL; // 节点不存在,返回 NULL
}
}
```
此函数从链表的头节点开始,逐个访问直到找到第 i 个节点,如果找到了则返回当前节点指针,否则返回 NULL。
(2) 求最大值函数 max (遍历单链表求最大值)
```c
int max(Node* head) {
if (head == NULL) {
return INT_MIN; // 空链表,返回最小整数值防止溢出
}
int max_val = head->data;
Node* current = head->next;
while (current != NULL) {
if (current->data > max_val) {
max_val = current->data;
}
current = current->next;
}
return max_val;
}
```
此函数初始化最大值为头节点的数据,然后遍历整个链表,每次遇到比当前最大值更大的节点就更新最大值,最终返回整个链表的最大值。
阅读全文
相关推荐
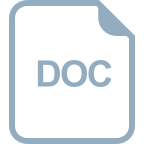
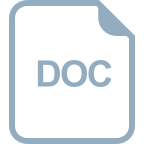
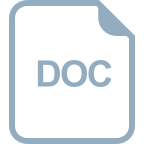

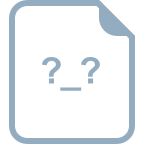
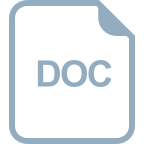
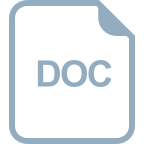
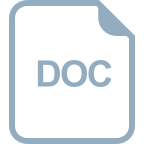
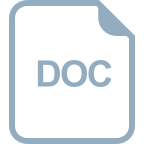
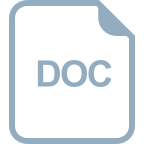

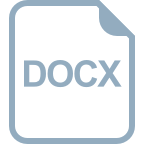
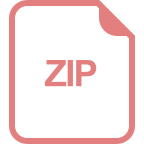