用之前学习的顺序表定义方法,编写多个数据(至少十个),用折半查找方法,查找顺序表中是否有自己想要查找的数据。C++
时间: 2024-10-07 10:04:23 浏览: 25
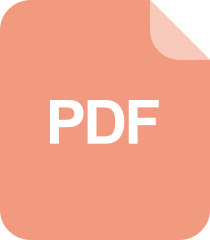
C语言实现顺序表的顺序查找和折半查找
在C++中,我们可以首先定义一个顺序表结构,然后实现一个折半查找算法。以下是这个过程的一个简单示例:
```cpp
#include <iostream>
using namespace std;
// 定义顺序表节点结构
struct ListNode {
int data;
ListNode* next;
ListNode(int val) : data(val), next(nullptr) {}
};
// 顺序表类,包含插入、删除和查找方法
class LinkedList {
private:
ListNode* head;
public:
LinkedList() : head(nullptr) {}
// 插入数据到列表尾部
void insert(int value) {
ListNode* newNode = new ListNode(value);
if (!head) {
head = newNode;
} else {
ListNode* current = head;
while (current->next) {
current = current->next;
}
current->next = newNode;
}
}
// 折半查找函数,返回值表示数据是否存在
bool binarySearch(int target) {
return search(head, target, 0, countElements());
}
private:
int countElements() {
int count = 0;
ListNode* temp = head;
while (temp) {
count++;
temp = temp->next;
}
return count;
}
// 实现折半查找的具体逻辑
bool search(ListNode* start, int target, int low, int high) {
if (high >= low) {
int mid = (low + high) / 2;
if (start[mid].data == target) {
return true; // 找到了目标
} else if (start[mid].data > target) {
return search(start, target, low, mid - 1); // 搜索左半部分
} else {
return search(start, target, mid + 1, high); // 搜索右半部分
}
}
return false; // 数据不存在
}
};
int main() {
LinkedList list;
for (int i = 0; i < 10; i++) { // 插入10个数据
list.insert(i);
}
// 使用折半查找功能
int target = 5;
bool found = list.binarySearch(target);
if (found) {
cout << "找到了数字" << target << endl;
} else {
cout << "未找到数字" << target << endl;
}
return 0;
}
```
在这个例子中,我们首先创建了一个`LinkedList`类,包含一个指向头节点的指针。接着,我们实现了插入和折半查找的功能。在`main()`函数中,我们创建了一个顺序表并插入了10个数据,然后使用折半查找寻找指定的目标值。
阅读全文
相关推荐
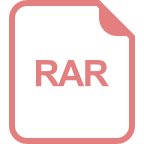
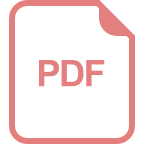












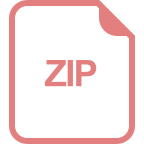