C#中.NET Framework4.5 https GET 带Header参数
时间: 2024-09-27 14:12:23 浏览: 7
在C#中,如果你想要使用.NET Framework 4.5发送HTTPS GET请求并带Header参数,你可以使用HttpClient类。以下是一个示例:
```csharp
using System;
using System.Net.Http;
using System.Net.Http.Headers;
namespace YourNamespace
{
class Program
{
static void Main(string[] args)
{
// 创建HttpClient实例
using (HttpClient client = new HttpClient())
{
// 设置请求的URL
string url = "https://example.com/api/resource";
// 定义Header
var headers = new Dictionary<string, string>
{
{ "Content-Type", "application/json" },
{ "Authorization", "Bearer your_token_here" } // 示例头,替换为你实际需要的值
};
// 使用UriBuilder构建完整的URL,如果包含查询字符串
if (!string.IsNullOrEmpty(url.Contains("?")))
{
url += UriQueryBuilder.EncodeQuery(headers);
}
// 创建请求消息
HttpRequestMessage request = new HttpRequestMessage(HttpMethod.Get, url);
// 添加Header到请求中
foreach (KeyValuePair<string, string> header in headers)
{
request.Headers.Add(header.Key, header.Value);
}
try
{
// 发送GET请求
HttpResponseMessage response = await client.SendAsync(request);
// 检查响应状态码
if (response.IsSuccessStatusCode)
{
Console.WriteLine(await response.Content.ReadAsStringAsync());
}
else
{
Console.WriteLine($"Error: {response.StatusCode}");
}
}
catch (Exception ex)
{
Console.WriteLine($"Error sending request: {ex.Message}");
}
}
}
// 将Header添加到URL的查询字符串中,用于处理复杂情况
private static string UriQueryBuilderEncodeQuery(Dictionary<string, string> headers)
{
StringBuilder sb = new StringBuilder();
bool first = true;
foreach (KeyValuePair<string, string> header in headers)
{
if (first)
first = false;
else
sb.Append("&");
sb.AppendFormat("{0}={1}", Uri.EscapeDataString(header.Key), Uri.EscapeDataString(header.Value));
}
return sb.ToString();
}
}
}
```
相关推荐
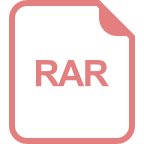
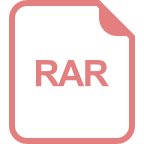
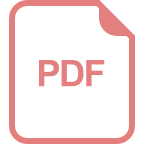



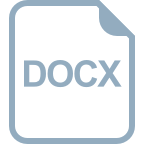
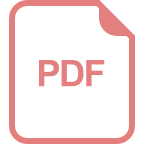
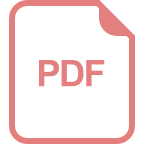
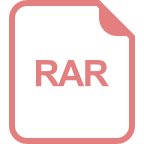
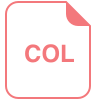
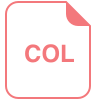
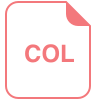
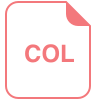
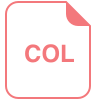
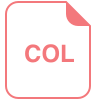
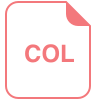
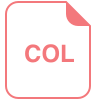