std::string类型转double类型
时间: 2024-10-16 10:14:16 浏览: 23
`std::string` 类型的字符串转换为 `double` 类型通常涉及到使用 `std::stod` 或者 `std::stof` 函数。这两个函数分别用于处理十进制和浮点数字符串。
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "3.14";
double num = std::stod(str);
std::cout << "Converted double value: " << num << std::endl;
return 0;
}
```
在这个例子中,`stod` 函数将字符串 "3.14" 转换为对应的 `double` 值 3.14。
如果字符串无法直接转换为数字(比如包含非数字字符),这些函数会抛出异常。为了安全处理这种情况,你可以使用 `try-catch` 结构:
```cpp
std::string str = "not a number";
try {
double num = std::stod(str);
// handle successful conversion
} catch (const std::invalid_argument& e) {
// handle invalid input
std::cerr << "Invalid number format: " << str << std::endl;
}
```
相关问题
std::basic_string可以使用std::to_string转换成std::string吗
`std::basic_string<CharT>` 类型(例如 `std::basic_string<char>` 或其他内置字符类型),本身是可以直接转换为 `std::string` 的,因为 `std::string` 可以视为 `std::basic_string<char>` 的特例。你可以通过 `std::string` 的构造函数将 `std::basic_string<CharT>` 的内容复制到一个新的 `std::string` 中。比如:
```cpp
std::basic_string<char> str = "Hello";
std::string strAsStdString = str; // 直接转换
```
当然,如果 `std::basic_string<CharT>` 实际上存储的是非ASCII字符,而你需要将其转换为 `std::string`,那么 `std::to_string` 就不适用了,因为它只针对 `int`、`double` 等数值类型。在这种情况下,你可以先将其内容转换为 `std::wstring`,然后再转为 `std::string`,利用 `std::wstring_convert` 或者 `std::codecvt_utf8_utf16`。
D:\work\f1_test\build-F1-Replacement_for_Desktop_Qt_5_15_2_MSVC2019_64bit-Debug\vcpkg_installed\x64-windows\include\nlohmann\detail\conversions\from_json.hpp:329: error: C2280: 'std::pair<const std::string,double> &std::pair<const std::string,double>::operator =(volatile const std::pair<const std::string,double> &)': attempting to reference a deleted function D:\work\f1_test\build-F1-Replacement_for_Desktop_Qt_5_15_2_MSVC2019_64bit-Debug\vcpkg_installed\x64-windows\include\nlohmann/detail/conversions/from_json.hpp(329): error C2280: 'std::pair<const std::string,double> &std::pair<const std::string,double>::operator =(volatile const std::pair<const std::string,double> &)': attempting to reference a deleted function D:\vs2019\2019install\VC\Tools\MSVC\14.29.30133\include\utility(269): note: see declaration of 'std::pair<const std::string,double>::operator =' D:\vs2019\2019install\VC\Tools\MSVC\14.29.30133\include\utility(269): note: 'std::pair<const std::string,double> &std::pair<const std::string,double>::operator =(volatile const std::pair<const std::string,double> &)': function was explicitly deleted D:\work\f1_test\build-F1-Replacement_for_Desktop_Qt_5_15_2_MSVC2019_64bit-Debug\vcpkg_installed\x64-windows\include\nlohmann/detail/conversions/from_json.hpp(391): note: see reference to function template instantiation 'void nlohmann::detail::from_json<BasicJsonType,const std::string,double>(const BasicJsonType &,std::pair<const std::string,double> &)' being compiled
这是一段编译错误信息,看起来是在使用nlohmann库中的from_json函数时发生了错误。具体来说,是在尝试给一个被声明为不可复制的const std::pair<const std::string,double>类型对象赋值时出现了问题。这可能与编译器版本、代码实现方式或使用的库版本有关。建议检查代码中对该对象的使用,以及相关库的版本和使用方式,以解决此问题。
阅读全文
相关推荐
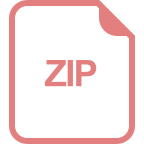
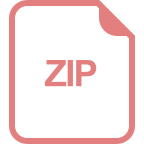
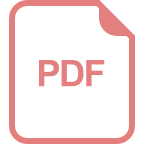









