IIC Verilog
时间: 2023-09-02 14:09:26 浏览: 64
IIC (Inter-Integrated Circuit) is a communication protocol commonly used for connecting devices in a master-slave configuration. In Verilog, you can design and implement I2C functionality using the language constructs.
To implement I2C in Verilog, you need to define the I2C module, including the input and output ports for SDA (Serial Data) and SCL (Serial Clock) lines. You also need to define internal registers and logic to handle the I2C protocol.
Here is an example of a basic I2C module in Verilog:
```verilog
module I2C (
input wire clk,
input wire rst,
inout wire sda,
inout wire scl
);
reg [7:0] i2c_data;
reg i2c_start;
reg i2c_stop;
// I2C state machine (implementing the protocol)
reg [2:0] state;
always @(posedge clk or posedge rst) begin
if (rst) begin
// Reset signals and state
sda <= 1'b1;
scl <= 1'b1;
i2c_start <= 1'b0;
i2c_stop <= 1'b0;
i2c_data <= 8'b0;
state <= 3'b000;
end else begin
case (state)
// I2C start condition
3'b000: begin
sda <= 1'b0;
scl <= 1'b0;
i2c_start <= 1'b1;
state <= 3'b001;
end
// I2C data transfer
3'b001: begin
// Implement data transfer logic here
// ...
end
// I2C stop condition
3'b010: begin
sda <= 1'b0;
scl <= 1'b1;
i2c_stop <= 1'b1;
state <= 3'b011;
end
// I2C idle state
3'b011: begin
sda <= 1'b1;
scl <= 1'b1;
i2c_start <= 1'b0;
i2c_stop <= 1'b0;
i2c_data <= 8'b0;
state <= 3'b000;
end
endcase
end
end
// Other implementation details go here...
endmodule
```
Please note that this is just a basic example to demonstrate the structure of an I2C module in Verilog. You will need to add appropriate logic to handle data transfer, acknowledge signals, and error conditions as per your specific requirements.
相关推荐
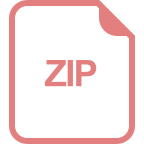














