iic verilog
时间: 2023-09-11 22:08:06 浏览: 128
IIC (Inter-Integrated Circuit) is a serial communication protocol used to connect low-speed peripherals with microcontrollers or other devices. Verilog is a hardware description language used to design digital circuits.
To implement IIC protocol in Verilog, you need to define the IIC module with input and output ports for data and clock lines. The module should include a state machine that controls the start, stop, and data transfer operations of the IIC bus. Here is an example code for IIC master module in Verilog:
```
module iic_master(
input wire clk,
input wire rst,
input wire [7:0] addr,
input wire [7:0] data_in,
input wire wr_en,
output reg [7:0] data_out,
output reg sda,
output reg scl
);
reg [7:0] data_buf;
reg [3:0] state;
parameter IDLE = 4'b0000;
parameter START = 4'b0001;
parameter ADDR = 4'b0010;
parameter DATA = 4'b0100;
parameter STOP = 4'b1000;
always @(posedge clk, posedge rst)
begin
if (rst) begin
state <= IDLE;
sda <= 1'b1;
scl <= 1'b1;
end
else begin
case (state)
IDLE: begin
if (wr_en) begin
data_buf <= data_in;
state <= START;
end
else begin
data_out <= 8'h00;
state <= IDLE;
end
end
START: begin
sda <= 1'b0;
scl <= 1'b0;
state <= ADDR;
end
ADDR: begin
for (i=0; i<8; i=i+1) begin
sda <= addr[7-i];
scl <= 1'b1;
scl <= 1'b0;
end
sda <= 1'b1;
scl <= 1'b1;
state <= DATA;
end
DATA: begin
for (i=0; i<8; i=i+1) begin
sda <= data_buf[7-i];
scl <= 1'b1;
scl <= 1'b0;
end
sda <= 1'b1;
scl <= 1'b1;
state <= STOP;
end
STOP: begin
sda <= 1'b0;
scl <= 1'b1;
sda <= 1'b1;
scl <= 1'b0;
state <= IDLE;
data_out <= data_in;
end
endcase
end
end
endmodule
```
This Verilog code defines an IIC master module that takes 8-bit address and data inputs and performs write operations to the IIC bus. The module includes a state machine that controls the start, address, data, and stop operations of the IIC bus. The code can be modified for different IIC operations and for slave mode operation.
阅读全文
相关推荐
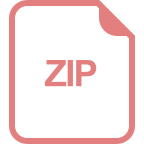
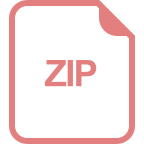
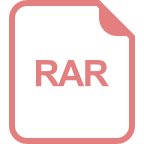
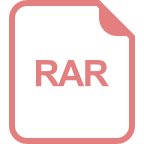

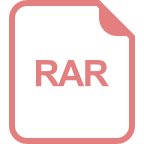
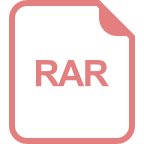
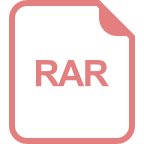
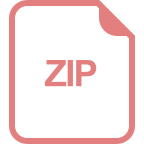
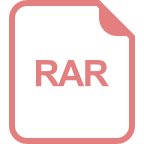
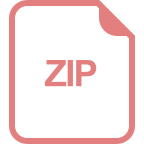
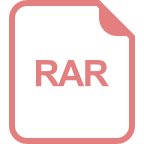
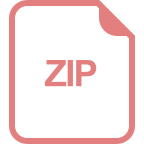
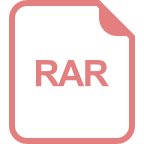
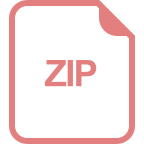