python pyqt计算利息
时间: 2023-11-28 22:44:06 浏览: 56
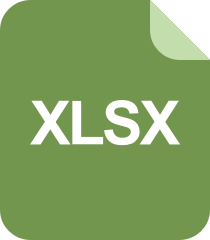
利息计算程序
以下是一个简单的Python PyQt5计算利息的例子:
```python
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QLineEdit, QPushButton
class InterestCalculator(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
# 设置窗口标题和大小
self.setWindowTitle('Interest Calculator')
self.setGeometry(300, 300, 300, 200)
# 创建标签和文本框
principalLabel = QLabel('Principal:', self)
principalLabel.move(20, 20)
self.principalEdit = QLineEdit(self)
self.principalEdit.move(120, 20)
rateLabel = QLabel('Rate:', self)
rateLabel.move(20, 60)
self.rateEdit = QLineEdit(self)
self.rateEdit.move(120, 60)
timeLabel = QLabel('Time:', self)
timeLabel.move(20, 100)
self.timeEdit = QLineEdit(self)
self.timeEdit.move(120, 100)
resultLabel = QLabel('Result:', self)
resultLabel.move(20, 140)
self.resultLabel = QLabel('', self)
self.resultLabel.move(120, 140)
# 创建按钮并绑定事件
calculateButton = QPushButton('Calculate', self)
calculateButton.move(200, 170)
calculateButton.clicked.connect(self.calculateInterest)
def calculateInterest(self):
# 获取输入的本金、利率和时间
principal = float(self.principalEdit.text())
rate = float(self.rateEdit.text())
time = float(self.timeEdit.text())
# 计算复利
interest = principal * (1 + rate / 100) ** time - principal
# 显示结果
self.resultLabel.setText(str(interest))
if __name__ == '__main__':
app = QApplication(sys.argv)
calculator = InterestCalculator()
calculator.show()
sys.exit(app.exec_())
```
这个例子创建了一个简单的GUI窗口,用户可以输入本金、利率和时间,然后点击“Calculate”按钮计算复利。计算结果将显示在窗口中。
阅读全文
相关推荐
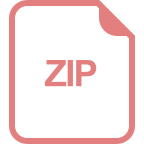
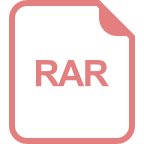
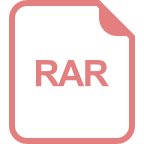
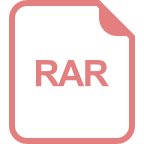
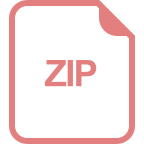
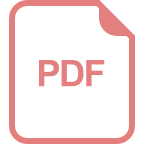
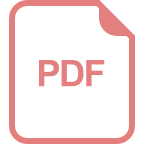
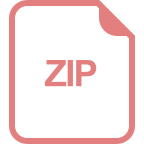
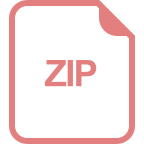
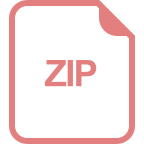
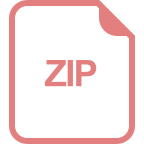
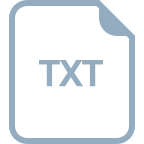
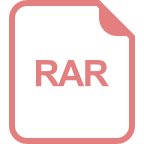