fastapi和异步
时间: 2024-04-18 10:20:19 浏览: 11
FastAPI是一个基于Python的现代、快速(高性能)的Web框架,用于构建API。它具有简单易用的语法和强大的功能,可以帮助开发者快速构建高性能的Web应用程序。
异步编程是一种编程模型,它允许程序在执行某些耗时操作时不会被阻塞,而是可以继续执行其他任务。在传统的同步编程中,当程序执行一个耗时操作时,它会一直等待操作完成后才能继续执行下一个任务。而异步编程通过使用异步函数和协程来实现非阻塞的操作,提高了程序的并发性和响应性能。
FastAPI与异步编程结合使用,可以充分利用Python的异步特性,提供高性能的Web服务。FastAPI使用了基于异步的框架Starlette和异步HTTP客户端httpx,可以处理大量并发请求,并且支持异步数据库访问、异步任务处理等功能。
总结一下,FastAPI是一个基于Python的高性能Web框架,通过与异步编程结合使用,可以实现非阻塞的操作和高并发的处理能力。
相关问题
fastapi sqlalchemy 异步
FastAPI是一个现代的Web框架,它支持异步编程和SQLAlchemy ORM。使用FastAPI和SQLAlchemy ORM可以轻松地构建高性能的Web应用程序。
在FastAPI中使用SQLAlchemy ORM时,可以使用asyncio和async/await语法来实现异步操作。这样可以提高应用程序的性能和吞吐量。
要在FastAPI中使用SQLAlchemy ORM,需要安装SQLAlchemy和asyncpg(或其他支持异步PostgreSQL的库)。然后,可以使用SQLAlchemy的ORM来定义模型,并使用async/await语法来执行数据库操作。
以下是一个使用FastAPI和SQLAlchemy ORM的示例:
```python
from fastapi import FastAPI
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
app = FastAPI()
SQLALCHEMY_DATABASE_URL = "postgresql://user:password@localhost/dbname"
engine = create_engine(SQLALCHEMY_DATABASE_URL)
SessionLocal = sessionmaker(autocommit=False, autoflush=False, bind=engine)
Base = declarative_base()
class User(Base):
__tablename__ = "users"
id = Column(Integer, primary_key=True, index=True)
name = Column(String)
email = Column(String, unique=True)
@app.post("/users/")
async def create_user(name: str, email: str):
db = SessionLocal()
user = User(name=name, email=email)
db.add(user)
db.commit()
db.refresh(user)
return user
```
在上面的示例中,我们定义了一个User模型,并在create_user函数中使用async/await语法来执行数据库操作。我们使用SessionLocal来创建数据库会话,并使用add方法将新用户添加到数据库中。
fastapi 异步调用
使用 FastAPI 进行异步调用需要使用 Python 的异步特性,例如使用 async/await 关键字和 asyncio 库。以下是使用 FastAPI 进行异步调用的示例代码:
```python
from fastapi import FastAPI
from fastapi.responses import HTMLResponse
import httpx
app = FastAPI()
@app.get("/", response_class=HTMLResponse)
async def root():
async with httpx.AsyncClient() as client:
response = await client.get("https://www.example.com")
return response.text
```
在这个示例中,我们使用了 httpx 库进行异步 HTTP 请求,并在 FastAPI 的路由函数中使用了 async/await 关键字。为了启用异步支持,我们将返回的响应类型设置为 HTMLResponse,并将函数定义为 async。
除了使用异步库外,还可以使用 FastAPI 内置的异步支持。例如,使用 asyncpg 库进行异步 PostgreSQL 数据库访问的示例代码如下:
```python
import asyncpg
from fastapi import FastAPI
app = FastAPI()
@app.on_event("startup")
async def startup():
app.state.pool = await asyncpg.create_pool("postgresql://user:password@localhost/dbname")
@app.get("/")
async def read_root():
async with app.state.pool.acquire() as connection:
result = await connection.fetch("SELECT 1")
return {"result": result}
```
在这个示例中,我们在 FastAPI 的应用程序启动事件中创建了一个异步 PostgreSQL 连接池,并在路由函数中使用了 async/await 关键字进行异步查询。
相关推荐
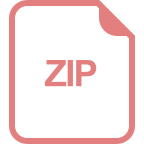
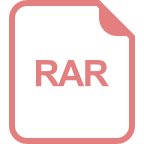
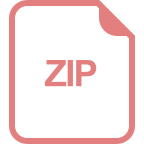






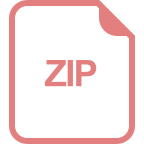
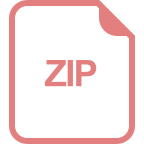
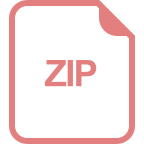
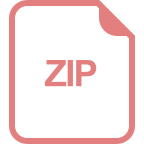
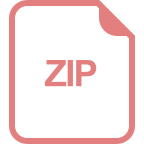
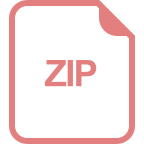
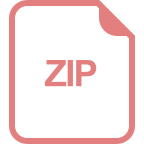