构建MLP,实现手写数字识别任务的代码
时间: 2024-11-27 15:20:43 浏览: 21
构建一个简单的多层感知机(MLP)用于手写数字识别通常涉及到以下步骤,这里以TensorFlow库为例:
首先,你需要安装必要的库:
```bash
pip install tensorflow numpy pandas sklearn
```
然后,使用Keras API创建一个基本的MLP模型:
```python
import tensorflow as tf
from tensorflow.keras import layers
from tensorflow.keras.datasets import mnist
from sklearn.model_selection import train_test_split
# 加载MNIST数据集
(x_train, y_train), (x_test, y_test) = mnist.load_data()
# 数据预处理
x_train = x_train.reshape(-1, 28 * 28) / 255.
x_test = x_test.reshape(-1, 28 * 28) / 255.
y_train = tf.keras.utils.to_categorical(y_train, num_classes=10)
y_test = tf.keras.utils.to_categorical(y_test, num_classes=10)
# 划分训练集和验证集
x_train, x_val, y_train, y_val = train_test_split(x_train, y_train, test_size=0.2, random_state=42)
# 定义MLP模型
model = tf.keras.Sequential([
layers.Dense(256, activation='relu', input_shape=(28 * 28,)), # 输入层
layers.Dropout(0.2), # 防止过拟合
layers.Dense(128, activation='relu'),
layers.Dropout(0.2),
layers.Dense(10, activation='softmax') # 输出层
])
# 编译模型
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# 训练模型
history = model.fit(x_train, y_train, validation_data=(x_val, y_val), epochs=10, batch_size=128)
# 评估模型
test_loss, test_acc = model.evaluate(x_test, y_test)
print(f"测试精度: {test_acc * 100:.2f}%")
# 相关问题--
1. 为什么在MLP中添加Dropout层?
2. 如何调整MLP的层数和节点数以优化性能?
3. 模型训练过程中如何防止过拟合?
阅读全文
相关推荐
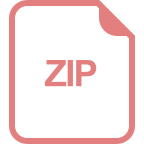
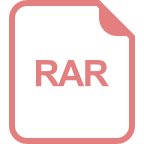
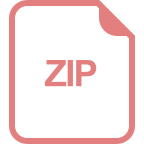

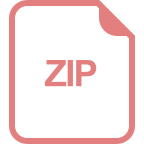
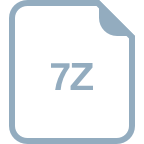
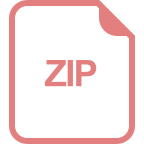
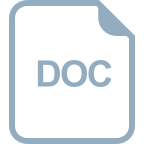
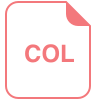




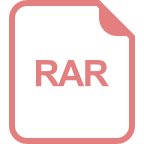
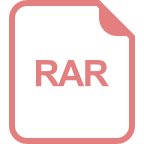
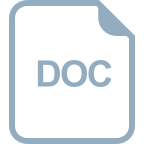
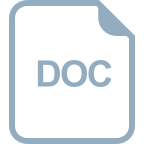


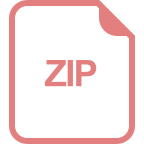