pyqt5 framelesswindowhint 鼠标无法缩放窗口
时间: 2024-02-03 21:00:46 浏览: 35
在使用PyQt5的FramelessWindowHint时,窗口是没有默认的边框和标题栏的,这意味着没有标准的鼠标操作来调整窗口的大小。但是,我们可以通过其他方式实现窗口大小的调整。
要实现窗口大小的调整,我们可以使用QWidget的鼠标事件以及setMouseTracking方法来捕捉鼠标的移动和点击事件,并根据这些事件的坐标来实时改变窗口的大小。
具体的实现步骤如下:
1. 创建一个继承自QWidget的自定义窗口类,并设置FramelessWindowHint标志。
2. 重写鼠标事件的方法:
- 重写mousePressEvent方法,捕捉鼠标按下事件,并记录下鼠标按下时的坐标。
- 重写mouseMoveEvent方法,捕捉鼠标移动事件,并计算出当前窗口在X轴和Y轴的大小改变量,并使用resize方法调整窗口的大小。
3. 在构造函数中调用setMouseTracking方法,设置为True,以确保鼠标跟踪事件得到捕捉。
这样,当鼠标按下并移动时,窗口大小会根据鼠标的移动而改变,实现了窗口的缩放效果。
需要注意的是,由于没有边框和标题栏,不能直接拖动窗口。如果需要实现窗口的拖动功能,可以在鼠标按下事件中记录下鼠标按下时的全局坐标,并使用窗口的move方法来改变窗口的位置。
希望以上内容对您有帮助。如有其他疑问,请随时提问。
相关问题
pyqt5 鼠标移动窗口
在PyQt5中,可以通过鼠标移动窗口来实现拖动窗口的功能。以下是实现这一功能的步骤:
1. 导入必要的模块:
```python
from PyQt5.QtWidgets import QApplication, QWidget
from PyQt5.QtCore import Qt
```
2. 创建一个继承自QWidget的窗口类:
```python
class MyWindow(QWidget):
def __init__(self):
super().__init__()
```
3. 重写窗口的鼠标按下、鼠标移动和鼠标释放事件:
```python
def mousePressEvent(self, event):
self.oldPos = event.globalPos()
def mouseMoveEvent(self, event):
delta = event.globalPos() - self.oldPos
self.move(self.x() + delta.x(), self.y() + delta.y())
self.oldPos = event.globalPos()
def mouseReleaseEvent(self, event):
pass
```
在鼠标按下事件中,记录下当前鼠标的位置。在鼠标移动事件中,计算鼠标移动的距离,并将窗口移动相应的距离。在鼠标释放事件中,不做任何处理。
4. 创建应用程序对象并显示窗口:
```python
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MyWindow()
window.show()
sys.exit(app.exec_())
```
这样,当你按住窗口的标题栏拖动时,窗口会跟随鼠标移动。
pyqt5窗口的缩放按钮设置
在PyQt5中,可以使用`setCornerWidget()`方法为窗口设置缩放按钮。具体步骤如下:
1. 导入所需模块:
```python
from PyQt5.QtWidgets import QMainWindow, QToolBar, QToolButton
from PyQt5.QtGui import QIcon
```
2. 在主窗口类中,创建一个工具栏对象:
```python
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建工具栏对象
self.toolbar = QToolBar()
self.addToolBar(self.toolbar)
```
3. 创建一个QToolButton对象,并将其添加到工具栏中。为了实现缩放功能,必须将该按钮的`setAutoRaise()`方法设置为`True`,并将其图标设置为缩放图标。
```python
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建工具栏对象
self.toolbar = QToolBar()
self.addToolBar(self.toolbar)
# 创建缩放按钮
zoom_button = QToolButton()
zoom_button.setAutoRaise(True) # 设置为自动升起
zoom_button.setIcon(QIcon("zoom.png")) # 设置缩放图标
self.toolbar.addWidget(zoom_button) # 添加到工具栏中
```
4. 最后,将该按钮设置为窗口的缩放按钮,使用窗口的`setCornerWidget()`方法即可:
```python
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建工具栏对象
self.toolbar = QToolBar()
self.addToolBar(self.toolbar)
# 创建缩放按钮
zoom_button = QToolButton()
zoom_button.setAutoRaise(True) # 设置为自动升起
zoom_button.setIcon(QIcon("zoom.png")) # 设置缩放图标
self.toolbar.addWidget(zoom_button) # 添加到工具栏中
# 设置缩放按钮
self.setCornerWidget(zoom_button)
```
完整示例代码如下:
```python
from PyQt5.QtWidgets import QMainWindow, QToolBar, QToolButton
from PyQt5.QtGui import QIcon
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建工具栏对象
self.toolbar = QToolBar()
self.addToolBar(self.toolbar)
# 创建缩放按钮
zoom_button = QToolButton()
zoom_button.setAutoRaise(True) # 设置为自动升起
zoom_button.setIcon(QIcon("zoom.png")) # 设置缩放图标
self.toolbar.addWidget(zoom_button) # 添加到工具栏中
# 设置缩放按钮
self.setCornerWidget(zoom_button)
```
其中,`zoom.png`为缩放图标的文件名。你可以使用你自己的图标文件来替换它。
相关推荐
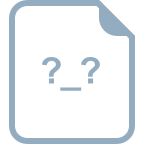











