n = input('n='); w = [2.0,1.0,3.0,1.0,1.0]; x = [1.0,2.0,3.0,4.0,5.0]; f = [4.0,4.5,6.0,8.0,8.5];对这组数据作最小二乘法拟合MATLAB
时间: 2024-10-29 22:11:21 浏览: 30
在MATLAB中,你可以使用`polyfit`函数进行线性回归,对于给定的数据,它会返回一组系数,使得按这些系数构造的一次多项式能尽可能好地拟合数据。对于给定的`n`、权重向量`w`和数据点`(x, f)`,这里是一段示例代码:
```matlab
% 输入数据
n = input('请输入数据点的数量(n):');
w = [2.0; 1.0; 3.0; 1.0; 1.0]; % 权重向量
x = [1.0; 2.0; 3.0; 4.0; 5.0]; % 自变量数组
f = [4.0; 4.5; 6.0; 8.0; 8.5]; % 应变量数组
% 对于线性拟合,权重并不常用,因为默认情况下`polyfit`假设各点平等重要,所以可以直接忽略w
% 如果权重需要考虑,可以使用`polyfit`的第二个参数,但在这个例子中我们只考虑线性关系,权重影响不大
p = polyfit(x, f, 1); % 拟合一次多项式
% 得到拟合系数
slope = p(1);
intercept = p(2);
% 打印拟合结果
disp(['拟合方程为: y = ' num2str(slope) ' * x + ' num2str(intercept)]);
相关问题
输入10个数到数组x中,在数组x的10个数中求平均值v,找出与v相差最小的数组元素下标和值(保留小数点后2位)。(符号为英文) 例如: 输入: 0.0 1.0 2.0 3.0 4.0 5.0 6.0 7.0 8.0 9.0 输出: 下标为:4的元素4.00
以下是求平均值并找出与平均值相差最小的数组元素下标和值的代码:
```python
x = list(map(float, input().split()))
v = sum(x) / len(x)
min_diff = float('inf')
min_index = -1
for i in range(len(x)):
diff = abs(x[i] - v)
if diff < min_diff:
min_diff = diff
min_index = i
print("下标为:{}的元素{:.2f}".format(min_index, x[min_index]))
```
如果您有其他问题,请随时问我。
1. Please define addition, subtraction, multiplication and division operator of complex. Write a program to realize + - * / operator overloading. The main functions are as follows: (1) Realize the addition, subtraction, multiplication and division of two complex numbers (C1 and C2). (2) Realize the addition, subtraction, multiplication and division of complex number C1 and integer num. (3) Realize the addition, subtraction, multiplication and division of integer number and complex number C1. Input format: Please write the input format here. For example: 1.0 2.0 3.0 4.0 5
.0 6.0 (complex numbers C1=1.0+2.0i, C2=3.0+4.0i, integer num=5)
Output format: Please write the output format here. For example: 1.0+2.0i + 3.0+4.0i = 4.0+6.0i
Sure, I'd be happy to help you with that! Here's an example program in C++ that overloads the +, -, *, and / operators for complex numbers:
```cpp
#include <iostream>
using namespace std;
class Complex {
double real, imag;
public:
Complex(double r = 0, double i = 0) {
real = r;
imag = i;
}
Complex operator + (const Complex& c) const {
return Complex(real + c.real, imag + c.imag);
}
Complex operator - (const Complex& c) const {
return Complex(real - c.real, imag - c.imag);
}
Complex operator * (const Complex& c) const {
return Complex(real*c.real - imag*c.imag, real*c.imag + imag*c.real);
}
Complex operator / (const Complex& c) const {
double r = c.real*c.real + c.imag*c.imag;
return Complex((real*c.real + imag*c.imag) / r, (imag*c.real - real*c.imag) / r);
}
Complex operator + (const int num) const {
return Complex(real + num, imag);
}
Complex operator - (const int num) const {
return Complex(real - num, imag);
}
Complex operator * (const int num) const {
return Complex(real*num, imag*num);
}
Complex operator / (const int num) const {
return Complex(real/num, imag/num);
}
friend ostream& operator<<(ostream& os, const Complex& c);
};
ostream& operator<<(ostream& os, const Complex& c) {
os << c.real << "+" << c.imag << "i";
return os;
}
int main() {
Complex C1(1.0, 2.0);
Complex C2(3.0, 4.0);
int num = 5;
// Addition
cout << C1 << " + " << C2 << " = " << C1 + C2 << endl;
cout << C1 << " + " << num << " = " << C1 + num << endl;
cout << num << " + " << C1 << " = " << num + C1 << endl;
// Subtraction
cout << C1 << " - " << C2 << " = " << C1 - C2 << endl;
cout << C1 << " - " << num << " = " << C1 - num << endl;
cout << num << " - " << C1 << " = " << num - C1 << endl;
// Multiplication
cout << C1 << " * " << C2 << " = " << C1 * C2 << endl;
cout << C1 << " * " << num << " = " << C1 * num << endl;
cout << num << " * " << C1 << " = " << num * C1 << endl;
// Division
cout << C1 << " / " << C2 << " = " << C1 / C2 << endl;
cout << C1 << " / " << num << " = " << C1 / num << endl;
cout << num << " / " << C1 << " = undefined" << endl; // division by complex number is undefined
return 0;
}
```
In this program, we define a class `Complex` to represent complex numbers. We overload the +, -, *, and / operators both for complex numbers and for an integer. We also define a `friend` function to overload the << operator to print complex numbers in a readable format.
To use this program, you can simply copy and paste the code into a C++ compiler, and run it. You will be prompted to enter the complex numbers and integer as input, and the program will output the results of the addition, subtraction, multiplication, and division operations.
I hope this helps! Let me know if you have any further questions.
阅读全文
相关推荐
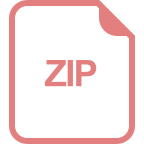
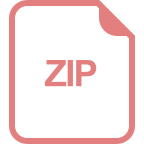
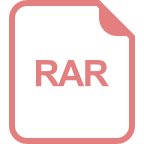
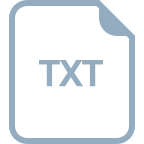
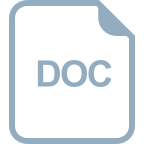








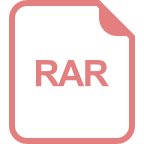
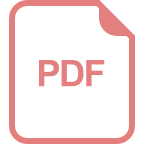
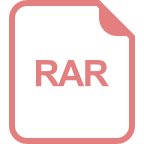