如何在Vue中实现审批流程的动态路由跳转?
时间: 2024-10-17 07:11:34 浏览: 33
在Vue中实现审批流程的动态路由跳转通常涉及到状态管理(如Vuex)和组件间的通信。以下是一个简单的步骤:
1. **状态管理** - 使用Vuex存储审批流程的状态,比如每个阶段、当前处理者等。你可以创建一个`approvalState`或类似的数据结构。
```js
// store/approval.js
export const state = () => ({
stages: ['initial', 'review', 'approved', 'rejected'],
currentStage: 'initial',
user: 'John Doe'
});
```
2. **路由配置** - 在`router/index.js`中为每个审批阶段设置对应的路由。使用动态参数来适应不同阶段:
```javascript
{
path: '/approval/:stage',
component: ApprovalComponent,
name: 'approval',
meta: { requiresAuth: true },
children: [
{
path: '',
component: StageComponent,
props: true // 配置props传递给组件
}
]
}
```
3. **组件设计** - 在`ApprovalComponent`中监听状态变化,根据`currentStage`动态渲染不同的子组件。例如,使用`v-if`或`v-show`:
```vue
<template>
<div>
<router-view :stage="currentStage"></router-view>
</div>
</template>
<script>
import { mapState } from "vuex";
export default {
computed: {
...mapState(['currentStage']) // 获取store中的currentStage
},
beforeRouteEnter(to, from, next) {
// 在进入组件之前确保用户已经登录
if (!to.meta.requiresAuth) return next();
// 检查是否允许进入当前阶段,根据实际情况编写逻辑
if (/* 用户有权限 */ && /* 当前阶段允许进入 */) {
next();
} else {
next(false); // 不加载组件,显示错误消息或者重定向到登录页面
}
}
};
</script>
```
4. **事件触发** - 在`StageComponent`中,处理审批操作(比如提交、驳回等),通过`this.$emit('update:stage', 新阶段)`来更新`currentStage`状态并触发父组件重新渲染:
```vue
<template>
...
<button @click="approve">批准</button>
...
</template>
<script>
export default {
methods: {
approve() {
this.$emit('update:stage', 'approved'); // 触发状态变更
}
}
};
</script>
```
阅读全文
相关推荐
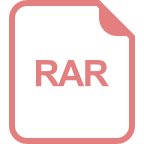
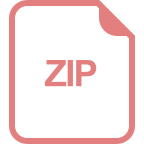
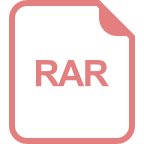
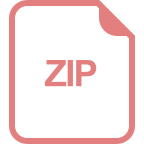
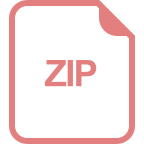
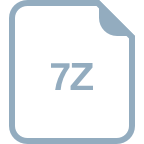
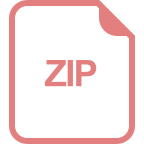
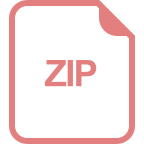
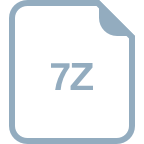
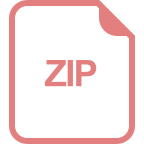
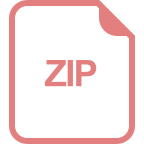
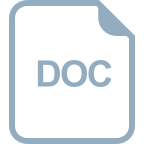
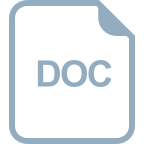




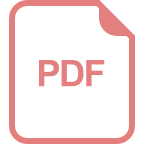