C语言程序是如何和串口建立联系
时间: 2024-09-06 17:04:50 浏览: 41
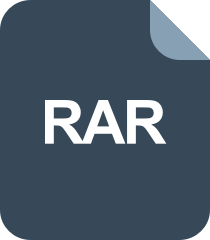
gpib_GPIB和串口_基于C语言的GPIB串口助手_

在C语言中,与串口(Serial Port)建立联系通常涉及到硬件的底层通信以及使用特定的库,例如POSIX标准提供的sys/time.h、fcntl.h、termios.h或者在Windows平台上使用Win32 API(如CreateFile、WriteFile、ReadFile等)。
以下是一个简单的步骤概述:
1. **包含头文件**:首先,你需要包含相关的头文件,如在Linux中可能会用到`#include <stdio.h>`(用于标准输入输出)、`#include <fcntl.h>`(文件描述符处理)、`#include <termios.h>`(控制终端)。
2. **打开串口**:使用`open()`函数,传入设备名(如`"/dev/ttyS0"` 或 `"/dev/ttyUSB0"`),设置访问权限和标志,以创建一个文件描述符。在Windows中,通常使用`CreateFile()`。
3. **配置串口**:使用`tcgetattr()`和`tcsetattr()`来获取和设置串口属性,如波特率、数据位、停止位和校验位等。在Linux中,`struct termios`结构体用于存储这些参数。
4. **读写操作**:通过文件描述符调用`read()`和`write()`函数进行数据发送和接收。在Windows上,对应的是`ReadFile()`和`WriteFile()`。
5. **关闭串口**:完成操作后,记得使用`close()`或`CloseHandle()`关闭打开的串口。
示例代码片段(简化版):
```c
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#include <termios.h>
int main() {
int fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY); // Linux
// or on Windows
// HANDLE hSerial = CreateFile("COM1", GENERIC_READ | GENERIC_WRITE, ...);
struct termios options;
tcgetattr(fd, &options);
// Set serial port configuration here...
cfsetispeed(&options, B9600); // 设置波特率为9600
options.c_cflag &= ~CSIZE; // Clear any existing size setting
options.c_cflag |= CS8; // Set 8 data bits
tcflush(fd, TCIFLUSH); // Flush input buffer
tcsetattr(fd, TCSANOW, &options); // Apply changes immediately
char send_data[] = "Hello from C!";
write(fd, send_data, strlen(send_data));
// Read from the serial port
char receive_data[100];
read(fd, receive_data, sizeof(receive_data));
printf("Received: %s\n", receive_data);
close(fd); // Close the device
return 0;
}
```
请注意,实际的代码可能会更复杂,特别是当你需要处理错误情况、同步读写或者异步通信的时候。在编写这类代码时,请确保理解你的目标平台及其特定的API。
阅读全文
相关推荐
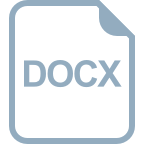
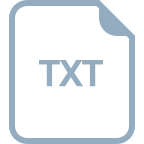
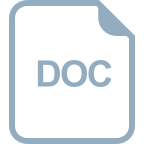
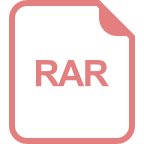
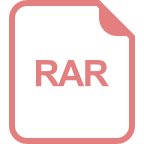
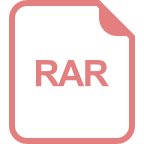
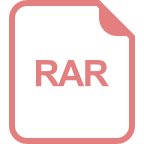
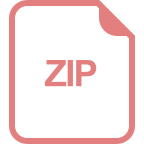
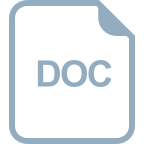
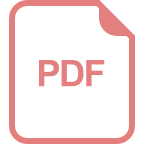
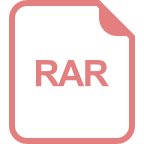
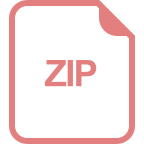
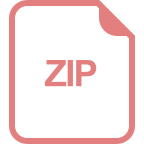
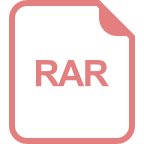
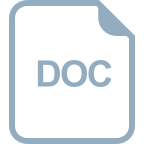
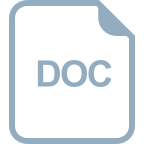
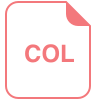
