如何使用Java POI库为Excel工作表中的特定单元格的左下角添加黄色的小三角标记?
时间: 2024-10-25 11:07:24 浏览: 15
在Java中,使用Apache POI库操作Excel时,为单元格添加图标标记通常需要通过`HSSFCellStyle`和`HSSFPatriarch`来实现。如果你想在单元格左下角添加一个小黄三角标记(条件格式的一种),可以按照以下步骤操作:
1. 导入所需的依赖:
```java
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
```
2. 创建一个新的Workbook实例,并选择XSSF格式,因为它支持更丰富的格式选项:
```java
Workbook workbook = new XSSFWorkbook();
```
3. 获取当前活跃的Sheet:
```java
Sheet sheet = workbook.createSheet("Sheet1");
```
4. 选择你想添加标记的单元格:
```java
Row row = sheet.createRow(0); // 行数从0开始
Cell cell = row.createCell(0); // 列数同样从0开始
```
5. 定义样式,包括填充颜色和图标:
```java
CellStyle style = workbook.createCellStyle();
DataFormat df = workbook.createDataFormat();
style.setFillForegroundColor(IndexedColors.YELLOW.getIndex());
style.setFillPattern(FillPatternType.SOLID_FOREGROUND);
// 添加条件格式规则,这里我们创建一个自定义的规则
Conditional FormattingRule rule = workbook.createConditionalFormattingRule();
rule.setRange(new CellRangeAddress(0, 0, 0, 0)); // 单元格范围
// 创建一个自定义的格式条件,比如基于公式
CellStyle triangleStyle = rule.create样式(); // 使用已定义的样式
FormulaEvaluator evaluator = sheet.getCreationHelper().createFormulaEvaluator();
Conditional FormattingThreshold threshold = rule.createThreshold();
threshold.setConditionType(CriteriaType.EQUAL_TO);
threshold.setValue((short) -1); // 比较值,这里设置为小于等于(-1代表TRUE)
threshold.setFormulaText("=" + "A1"); // 设置条件公式,如A1等于某个值才会应用黄色三角形
threshold.setCellStyle(triangleStyle); // 应用样式
rule AppliesToDataIs = rule.createAppliesToDataIs();
AppliesToDataIs.addCellRange(new CellRangeAddress("", "", "", "")); // 继续设置范围为所选单元格
rule.apply(sheet);
```
6. 将样式应用到单元格上:
```java
cell.setCellStyle(style);
```
7. 最后记得保存工作簿:
```java
FileOutputStream outputStream = new FileOutputStream("output.xlsx");
workbook.write(outputStream);
outputStream.close();
workbook.close();
```
阅读全文
相关推荐
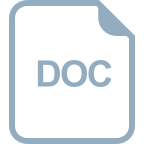
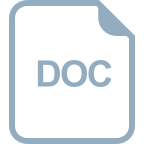
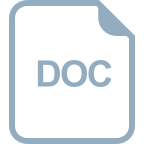
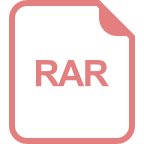
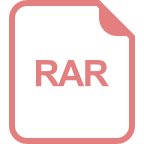
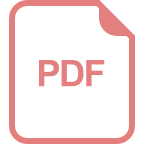
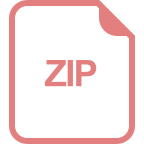
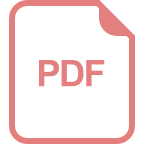
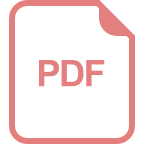
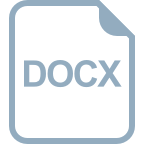
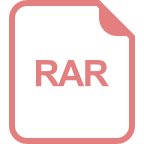
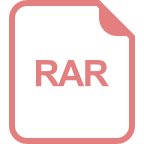
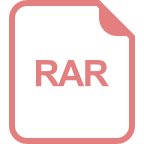
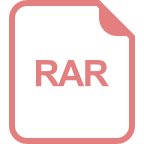
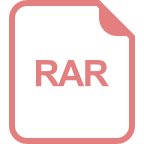
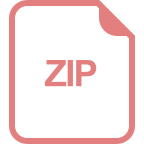
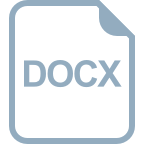
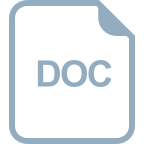