根据提示,使用 Python 实现梯度下降算法,并损失函数最小值时对应的参数theta,theta会返回给外部代码,由外部代码来判断theta是否正确。
时间: 2024-09-19 20:06:36 浏览: 46
梯度下降是一种常用的优化算法,用于寻找目标函数的局部最小值。在Python中,我们可以使用NumPy库来实现这个过程。首先,你需要定义一个假设的线性回归模型,比如我们有y = w * x + b这样的形式,其中w和b是我们需要找到的权重和偏置。
以下是一个基本的梯度下降实现示例:
```python
import numpy as np
def gradient_descent(x, y, learning_rate=0.01, num_iterations=1000):
# 初始化随机权重和偏置
theta = np.random.randn(2)
for i in range(num_iterations):
# 计算预测值和误差
hypothesis = np.dot(x, theta)
loss = hypothesis - y
# 梯度计算
grad = (1 / len(y)) * np.dot(x.T, loss)
# 更新参数
theta -= learning_rate * grad
return theta # 返回最终的theta参数
# 使用示例数据
x = np.array([[1], [2], [3], [4]])
y = np.array([2, 4, 6, 8]) # 假设这是y = x的数据
theta = gradient_descent(x, y)
print("最优解的参数 theta:", theta)
# 判断theta是否正确
# 这里你可以选择一个已知的标准答案或者使用其他方法评估theta的好坏
is_correct = check_theta(theta, x, y) # 自定义检查函数
if is_correct:
print("Theta看起来是正确的")
else:
print("Theta可能不是最佳解")
#
阅读全文
相关推荐
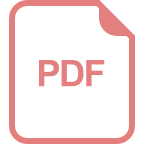
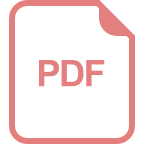
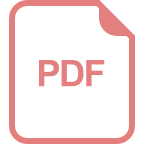

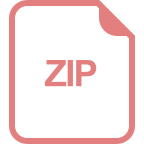
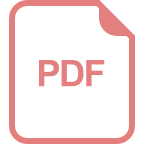
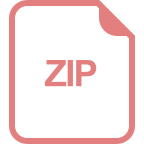
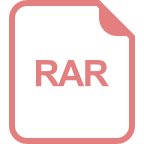
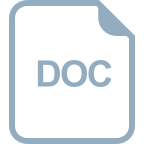
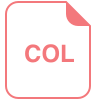
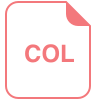







