用c语言实现顺序表的输入和输出
时间: 2024-10-14 08:07:14 浏览: 20
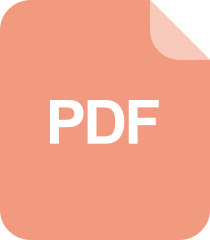
c语言实现顺序表的基本操作
在C语言中,我们可以使用数组来实现一个简单的顺序表,并提供相应的输入(insertion)和输出(display)操作。下面是一个基础的顺序表结构和函数示例:
```c
#include <stdio.h>
// 定义顺序表节点结构
typedef struct {
int data; // 数据域存储元素值
struct Node* next; // 指针域指向下一个元素
} Node;
// 初始化顺序表的第一个节点
Node* createList() {
Node* head = (Node*)malloc(sizeof(Node));
if (head == NULL) {
printf("Memory allocation failed.\n");
return NULL;
}
head->data = -1; // 空列表标记
head->next = NULL;
return head;
}
// 在列表末尾插入元素
void insert(int value, Node** head) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode == NULL) {
printf("Memory allocation failed.\n");
return;
}
newNode->data = value;
newNode->next = *head;
*head = newNode;
}
// 打印顺序表中的元素
void display(Node* head) {
Node* current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
int main() {
Node* list = createList();
// 输入并插入数据
int num;
printf("Enter elements to insert (or -1 to stop):\n");
scanf("%d", &num);
while (num != -1) {
insert(num, &list);
scanf("%d", &num);
}
// 输出显示
printf("Elements in the list:\n");
display(list);
free(list); // 释放内存
return 0;
}
```
在这个例子中,`createList()` 函数创建了一个空的顺序表,`insert()` 在列表末尾添加新元素,`display()` 则遍历列表并打印所有元素。用户可以按照提示逐个输入元素,程序会在结束后显示整个列表。
阅读全文
相关推荐
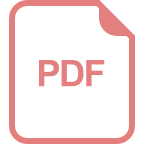
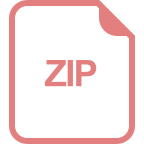
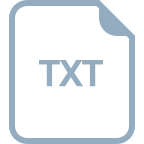
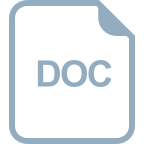
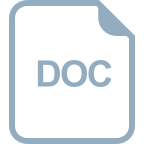
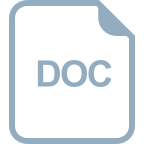
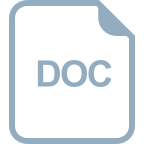
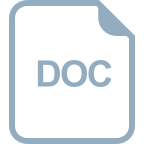
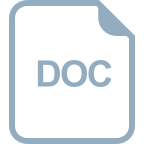
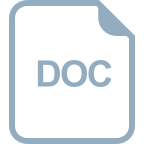




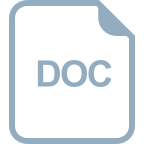
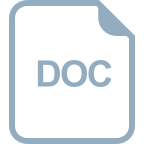
