pyqt5图书馆管理系统
时间: 2024-06-18 07:01:49 浏览: 18
PyQt5是一个强大的Python绑定库,它允许开发者使用Python语言来创建跨平台的应用程序,尤其是那些基于Qt库的图形用户界面(GUI)。一个图书馆管理系统(Library Management System, LMS)用PyQt5开发的话,可以包含以下主要功能:
1. **图书管理**:用户能够添加、删除和搜索图书信息,包括书名、作者、ISBN、分类等。
2. **借阅/归还**:跟踪图书的借阅状态,包括借阅日期、到期日期,并支持过期提醒或罚款计算。
3. **用户管理**:注册新用户,管理用户资料,如账户、借阅历史和权限等级。
4. **馆藏管理**:库存控制,包括添加、更新和删除图书资源。
5. **搜索功能**:支持多种搜索条件,如快速检索、模糊搜索等。
6. **报告和统计**:生成各类统计报表,如借阅量、热门书籍、滞纳金收入等。
使用PyQt5可以提供直观的图形界面,使得操作更加便捷。要开发这样的系统,你会用到PyQt5的QWidgets模块创建窗口和控件,信号槽机制处理事件,以及数据库操作(如SQLAlchemy或PyQtSql)来存储数据。
相关问题
pyqt5图书管理系统
PyQt5是一款用于创建用户界面的Python GUI库,它可以与Qt的C++库结合使用。图书管理系统是一种用于管理图书信息、读者信息及图书借阅信息的管理系统。将二者结合起来,就可以创建一款PyQt5图书管理系统。
使用PyQt5可以轻松地创建具有良好用户体验的图书管理系统,系统界面美观、交互式强,可以满足用户需求。除此之外,PyQt5还提供了大量丰富的组件,如标签、按钮、表格等,方便用户快速完成界面设计。同时PyQt5支持事件机制,可以方便地实现交互逻辑的编写。
在图书管理系统中,可以使用PyQt5完成以下功能:读者信息管理、图书信息管理、借阅信息管理、图书检索、提醒及催还等。具体来说,可以通过PyQt5创建表格、编写按钮事件、实现对图书信息的添加、修改、删除、查看,实现对读者信息的管理等。
总之,PyQt5图书管理系统是一款非常实用和优秀的图书管理软件,它将PyQt5优秀的界面设计和丰富的组件功能与图书管理系统完美结合,方便用户完成图书管理工作。同时,PyQt5图书管理系统的设计与实现也是一个很好的Python GUI编程实践,可以有助于提高学习者的编程水平。
基于PyQT5的图书管理系统
基于PyQT5的图书管理系统是一个能够帮助学院图书信息管理工作系统化、规范化、自动化的系统。该系统主要由前台和后台两部分组成。前台部分使用PyQT5进行设计和布局,使用PyCharm中的Qt Designer进行绘制,然后使用PyQT5和qss文件来编写窗口的样式。后台逻辑和数据的处理是使用Python结合MySQL数据库以及线程的使用来进行处理。该系统的功能包括书籍推荐、系统公告的展示、维护借阅信息和图书信息、提供给用户和管理员之间的交流等。管理员和普通用户的功能不同,管理员可以对图书信息进行管理,而普通用户只能进行图书的查询和借阅。以下是该系统的一些特点和优势:
- 系统化、规范化、自动化的图书信息管理,提高学院图书管理效率。
- 使用PyQT5进行设计和布局,界面美观、易用。
- 使用Python结合MySQL数据库进行后台逻辑和数据处理,处理效率高。
- 提供书籍推荐和系统公告的展示,方便用户获取信息。
- 维护借阅信息和图书信息,方便管理员进行管理。
- 提供用户和管理员之间的交流,方便系统的升级和维护。
相关推荐
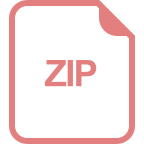
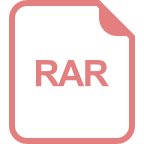
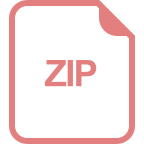
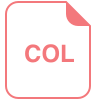
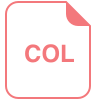
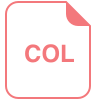
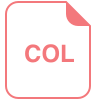
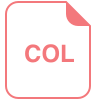








