如何使用for循环实现一个名为`longest`的方法,该方法接收一个包含字符串的ArrayList,目标是找到并返回列表中最长的字符串?如果存在多个相同长度的最长字符串,应返回最先出现的那个。请确保代码遵循这种方法论并且不使用内置函数来获取最长字符串。
时间: 2024-12-11 16:27:45 浏览: 13
为了实现这个名为 `longest` 的方法,你可以使用 for 循环遍历 ArrayList 中的每个元素,并维护一个变量(例如 `max_length` 和 `result`)来存储当前找到的最长字符串及其长度。以下是 Java 代码实现:
```java
import java.util.ArrayList;
public class Main {
public static String longest(ArrayList<String> stringList) {
if (stringList == null || stringList.isEmpty()) {
return null; // 如果列表为空,直接返回null
}
String result = stringList.get(0); // 初始假设第一个元素是最长的
int max_length = stringList.get(0).length();
// 遍历列表中的每一个字符串
for (int i = 1; i < stringList.size(); i++) {
String currentString = stringList.get(i);
int currentLength = currentString.length();
// 检查当前字符串是否更长
if (currentLength > max_length) {
result = currentString;
max_length = currentLength;
}
}
return result;
}
public static void main(String[] args) {
ArrayList<String> list = new ArrayList<>();
list.add("short");
list.add("very long string");
list.add("another short one");
System.out.println(longest(list)); // 输出:very long string
}
}
```
在这个例子中,我们首先检查输入列表是否为空。然后,从第一个元素开始比较,每次迭代都更新最长字符串和其长度。当遇到比当前最长字符串还要长的字符串时,就更新这两个值。最后,循环结束后,`result` 将保存最长的字符串。
阅读全文
相关推荐
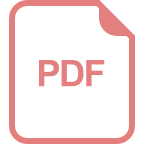
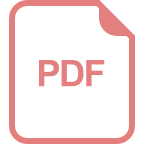
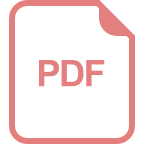


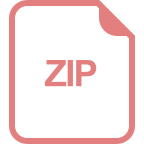
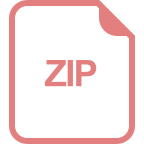











