用Python写一个拉普拉斯算子实现图像增强的程序
时间: 2024-10-03 07:04:58 浏览: 31
在Python中,我们可以使用PIL(Python Imaging Library)或者其升级版Pillow库以及numpy库来实现拉普拉斯算子进行图像增强。拉普拉斯算子是一种边缘检测算子,它可以突出图像中的高频信息,常用于图像处理中的噪声去除和边缘检测。
下面是一个简单的示例,使用PIL和numpy:
```python
from PIL import Image
import numpy as np
# 加载图像
img = Image.open('input_image.jpg')
img_array = np.array(img)
# 对图像数组应用拉普拉斯变换
laplacian = np.array([[0, 1, 0],
[1, -4, 1],
[0, 1, 0]]) # 拉普拉斯矩阵
laplacian_response = np.convolve(img_array, laplacian, mode='same') # 卷积操作
# 将结果转换回灰度图像
enhanced_img = laplacian_response / laplacian_response.max()
# 转换回PIL格式并保存
enhanced_img = Image.fromarray(enhanced_img.astype(np.uint8), 'L')
enhanced_img.save('enhanced_image.jpg')
相关问题
python拉普拉斯算子批量图像增强
作为一个AI语言模型,我可以提供相关的Python代码示例:
```
import cv2
import numpy as np
import os
# 拉普拉斯算子增强图像的函数
def laplacian_enhancement(image):
kernel = np.array([[0,-1,0],[-1,5,-1],[0,-1,0]])
dst = cv2.filter2D(image, -1, kernel)
return dst
# 批量处理图像的函数
def batch_process_images(src_path, dst_path):
if not os.path.exists(dst_path):
os.mkdir(dst_path)
for file_name in os.listdir(src_path):
if file_name.endswith('.jpg') or file_name.endswith('.png'):
file_path = os.path.join(src_path, file_name)
image = cv2.imread(file_path)
dst = laplacian_enhancement(image)
dst_file_path = os.path.join(dst_path, file_name)
cv2.imwrite(dst_file_path, dst)
# 测试
if __name__ == '__main__':
src_path = 'source_images'
dst_path = 'enhanced_images'
batch_process_images(src_path, dst_path)
```
以上代码实现了拉普拉斯算子批量图像增强的功能。具体实现步骤如下:
1. 定义 `laplacian_enhancement` 函数,实现拉普拉斯算子增强图像的功能。
2. 定义 `batch_process_images` 函数,实现批量处理图像的功能。该函数遍历源目录下的所有图像文件,对每个文件调用 `laplacian_enhancement` 函数进行图像增强,并将增强后的图像写入目标目录。
3. 在 `__main__` 函数中测试 `batch_process_images` 函数,将源目录和目标目录指定为 `source_images` 和 `enhanced_images`。
使用时需要将源图像放到 `source_images` 目录下,并确保该目录存在;程序将增强后的图像保存到 `enhanced_images` 目录中。
希望以上代码对你有帮助,如有疑问请随时提出。
编写一个Python程序。对图片做以下处理:加胡椒噪声,加盐粒噪声,最大值滤波,最小值滤波,高斯滤波,Sobel梯度算子,拉普拉斯算子,拉普拉斯锐化增强图像。把以上效果图在一张画布中显示出来。
编写一个Python程序,我们可以使用PIL(Python Imaging Library)库来进行基本的图像处理操作,以及OpenCV库来实现更复杂的滤波和边缘检测。首先确保已经安装了这两个库,如果没有可以使用`pip install pillow opencv-python-headless`命令安装。
以下是一个简单的示例代码,演示如何对图片进行上述处理:
```python
from PIL import Image, ImageFilter
import cv2
# 加载图片
image_path = 'your_image_path.jpg'
img_pil = Image.open(image_path)
img_cv = cv2.imread(image_path, cv2.IMREAD_GRAYSCALE) # 读取灰度图以便于后续处理
# 1. 加胡椒噪声 (Salt & Pepper Noise)
pepper_noise = img_pil.convert('L').point(lambda x: 0 if x < 128 else 255)
salt_noise = pepper_noise.point(lambda x: 255 if x < 128 else 0)
# 2. 最大值滤波 / 最小值滤波
max_filtered = img_pil.filter(ImageFilter.MaxFilter(size=3))
min_filtered = img_pil.filter(ImageFilter.MinFilter(size=3))
# 3. 高斯滤波
gaussian_filtered = img_pil.filter(ImageFilter.GaussianBlur(radius=3))
# 4. Sobel梯度算子 (OpenCV)
sobel_x = cv2.Sobel(img_cv, cv2.CV_64F, 1, 0, ksize=3)
sobel_y = cv2.Sobel(img_cv, cv2.CV_64F, 0, 1, ksize=3)
sobel_gradient = cv2.addWeighted(sobel_x, 0.5, sobel_y, 0.5, 0)
# 5. 拉普拉斯算子
laplacian = cv2.Laplacian(img_cv, cv2.CV_64F)
# 6. 拉普拉斯锐化 (增强)
laplacian_sharpened = img_cv + laplacian * 2
# 将所有处理后的图像合并到一个画布上
width = max(len(images) * images[0].width, image_pil.width)
height = sum(image.height for image in images) + 10 # 添加一些间距
combined_image = Image.new('RGB', (width, height), color=(255, 255, 255))
y_offset = 0
for image in [pepper_noise, salt_noise, max_filtered, min_filtered, gaussian_filtered,
sobel_gradient, laplacian_sharpened]:
combined_image.paste(image.resize((image.width, image.height)), (0, y_offset))
y_offset += image.height + 10 # 增加间距
# 显示原始和处理后的图像
combined_image.show()
# 输出结果保存
combined_image.save('processed_image.png')
阅读全文
相关推荐
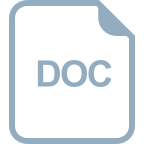
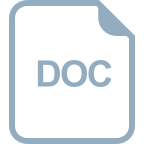
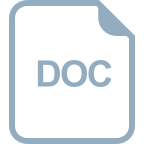
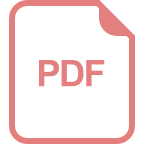
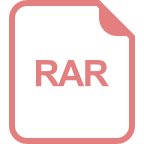

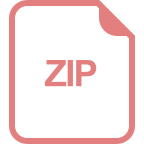
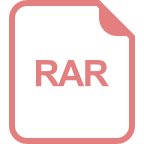
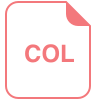
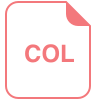

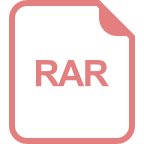
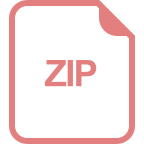
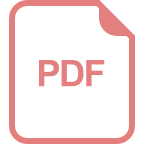
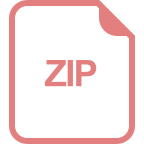
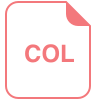
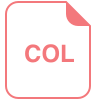