如何在C++中按升序/降序对一组数字进行排序?
时间: 2024-10-29 11:11:54 浏览: 21
在C++中,你可以使用标准库中的`std::sort()`函数来进行数组或容器的排序。这里以升序为例:
1. 对整数数组进行升序排序:
```cpp
#include <algorithm>
#include <vector>
int numbers[] = {5, 2, 8, 1, 9}; // 待排序的数组
std::vector<int> vec(numbers, numbers + sizeof(numbers) / sizeof(numbers[0])); // 转换为vector便于操作
// 使用std::sort(),第一个参数是范围的起始地址,第二个参数是结束地址(不包括)
std::sort(vec.begin(), vec.end());
// 或者直接排序原数组,注意这会改变原始数组
// std::sort(numbers, numbers + sizeof(numbers) / sizeof(numbers[0]));
```
2. 对自定义类型的元素进行升序排序,需要提供比较函数(如`std::greater<int>`用于降序):
```cpp
bool compare(int a, int b) {
return a < b; // 升序排序
// return a > b; // 如果想要降序排序
}
// 自定义类型的数组
Person people[] = {...};
// 使用lambda表达式传递比较函数给std::sort()
std::sort(people, people + sizeof(people) / sizeof(people[0]), compare);
```
对于降序排序,只需替换`<`为`>`即可。
阅读全文
相关推荐
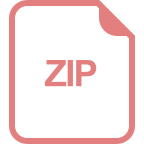
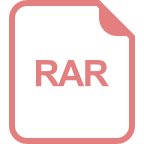
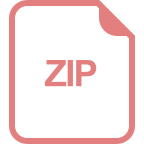















