用类模板完成如何实现两个单链表sLinkList对加法运算符的重载,使两个链表拼接起来
时间: 2024-10-14 18:07:28 浏览: 15
在C++中,为了实现两个单链表通过`+`运算符进行加法操作(即合并),我们可以利用类模板来创建一个通用的链表类,并对`operator+`进行重载。这里假设我们的链表节点包含一个整数值。
首先,我们需要定义一个基本的链表节点结构和链表类模板:
```cpp
template <typename T>
struct ListNode {
T value; // 节点值
ListNode* next; // 指向下一个节点的指针
};
template <typename T>
class LinkedList {
public:
// 构造函数
LinkedList() : head(nullptr) {}
// 链表节点添加方法
void addNode(T value) {
ListNode<T>* newNode = new ListNode<T>{value, nullptr};
newNode->next = head;
head = newNode;
}
// 用于重载的加法运算符
LinkedList<T>& operator+(const LinkedList<T>& other) {
// 先处理空链表的情况
if (this->head == nullptr) {
this->head = other.head;
} else if (other.head == nullptr) {
return *this; // 如果其他链表为空,直接返回当前链表
} else {
ListNode<T>* current = this->head;
while (current->next != nullptr && other.head != nullptr) {
current->next = current->next->next;
other.head = other.head->next;
}
if (other.head != nullptr) { // 将剩余的其他链表接到原链表的末尾
current->next = other.head;
}
}
return *this;
}
private:
ListNode<T>* head; // 链表头节点
};
```
现在,你可以使用`LinkedList<int>`来创建整数链表,并使用`+`操作符将它们合并:
```cpp
int list1[] = {1, 2, 3};
int list2[] = {4, 5, 6};
LinkedList<int> list1Instance(list1, sizeof(list1) / sizeof(list1[0]));
LinkedList<int> list2Instance(list2, sizeof(list2) / sizeof(list2[0]));
LinkedList<int> result = list1Instance + list2Instance;
// 现在result链表包含了list1和list2的内容:1, 2, 3, 4, 5, 6
```
阅读全文
相关推荐
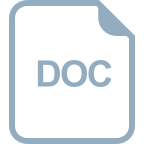
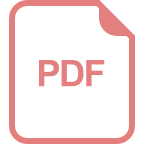
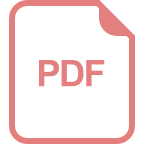



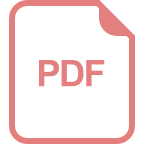
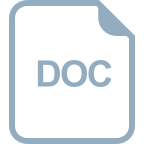
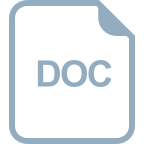
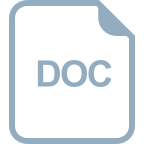
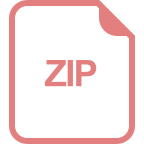
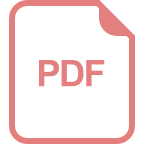
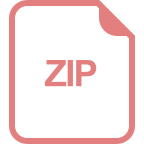