用类模板完成如何实现两个单链表sLinkList对加法运算符的重载,使两个链表拼接起来,请给出重载函数实现,其中应创建一个新的对象并返回这个对象
时间: 2024-10-14 16:07:35 浏览: 32
在C++中,我们可以使用类模板(Class Template)来实现通用的单链表加法运算符重载,这样不仅可以用于整数链表,也可以用于其他数据类型的链表。这里我们假设链表节点有一个公共的数据成员`data`和指向下一个节点的指针`next`。以下是实现的示例:
```cpp
template <typename T>
class Node {
public:
T data;
Node<T>* next;
// 构造函数
Node(T value = T()) : data(value), next(nullptr) {}
};
template <typename T>
class SLinkedList {
private:
Node<T>* head;
public:
// ... 其他链表操作函数 ...
// 重载加法运算符,合并两个链表
SLinkedList<T>& operator+(const SLinkedList<T>& other) {
if (this->head == nullptr) {
this->head = other.head;
} else {
Node<T>* current = this->head;
while (current->next != nullptr) {
current = current->next;
}
current->next = other.head;
}
return *this;
}
// 创建新的SLinkedList对象,包含当前链表的内容
SLinkedList<T> mergeLists() const {
SLinkedList<T> result(*this);
return result;
}
};
// 示例
int main() {
SLinkedList<int> list1 = {1, 2, 3};
SLinkedList<int> list2 = {4, 5};
SLinkedList<int> mergedList = list1 + list2;
// 或者使用mergeLists方法创建新链表
// SLinkedList<int> mergedList2 = list1.mergeLists();
return 0;
}
```
在这个例子中,`operator+`实现了链表的合并,而`mergeLists`函数则返回了一个新的`SLinkedList`对象,包含了原始链表的内容。这样当你对两个`SLinkedList`实例使用`+`操作时,会创建一个新的链表作为结果。
阅读全文
相关推荐
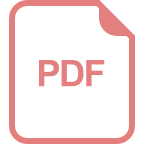
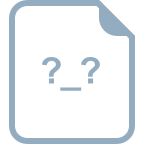
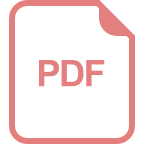


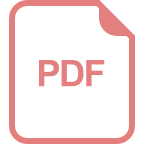
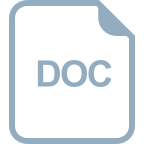
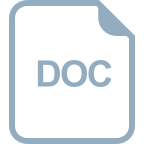
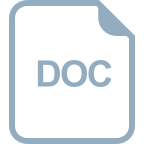
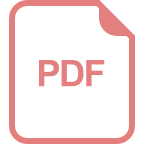
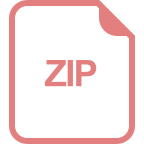
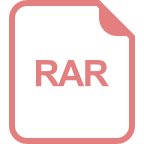
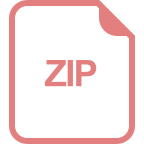
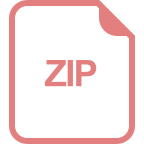
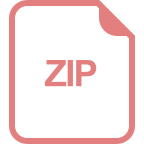
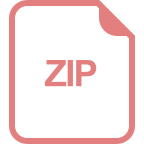
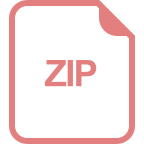
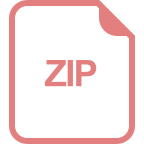