编写一个程序sqlist.cpp,实现顺序表的各种基本运算
时间: 2024-09-23 15:00:53 浏览: 23
编写一个名为`sqlist.cpp`的程序来实现顺序表(Sequential List)的基本运算,主要包括插入元素、删除元素、查找元素、以及打印列表等操作。顺序表是一种简单的线性数据结构,其中元素按照它们在数组中的位置存储。
以下是基本的顺序表类设计示例(假设使用C++):
```cpp
#include <iostream>
using namespace std;
// 定义顺序表节点
struct Node {
int data;
Node* next;
};
class SequentialList {
private:
Node* head; // 列表头指针
int size; // 列表长度
public:
// 构造函数
SequentialList() : head(nullptr), size(0) {}
// 插入元素到列表头部
void insertAtStart(int value) {
Node* newNode = new Node{value, head};
if (head)
head->next = newNode;
else
head = newNode;
size++;
}
// 删除指定值的第一个出现
void removeElement(int value) {
if (!head || !head->data == value)
return;
Node* temp = head;
head = head->next;
delete temp;
size--;
}
// 查找元素
bool findElement(int value) const {
Node* current = head;
while (current != nullptr) {
if (current->data == value)
return true;
current = current->next;
}
return false;
}
// 打印列表
void printList() const {
Node* temp = head;
while (temp != nullptr) {
cout << temp->data << " ";
temp = temp->next;
}
cout << endl;
}
// 获取列表大小
int getSize() const { return size; }
};
int main() {
SequentialList list;
list.insertAtStart(1);
list.insertAtStart(2);
list.insertAtStart(3);
list.printList(); // 输出 [3, 2, 1]
list.removeElement(2); // 删除第一个2
list.printList(); // 输出 [3, 1]
if (list.findElement(3)) {
cout << "Found 3 in the list" << endl;
} else {
cout << "3 not found in the list" << endl;
}
return 0;
}
```
这个程序展示了创建一个顺序表并执行一些基本操作的过程。注意这只是一个简化版本,实际项目中还需要考虑内存管理和其他错误处理情况。
相关推荐
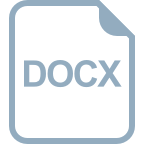
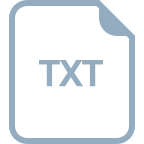
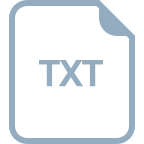















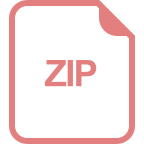