实验题1:实现顺序表的各种基本运算的算法 内容:编写一个程序 sqlist.Cpp
时间: 2024-09-25 07:10:03 浏览: 26
实验题1的目标是让你熟悉顺序表数据结构,并学习如何实现其基本操作,如插入、删除、查找以及显示元素等。以下是简要的步骤和伪代码描述:
```cpp
// 定义顺序表的基本结构体或类
struct ListNode {
int data;
ListNode* next; // 指向下一个节点的指针
};
class SequentialList {
public:
// 构造函数,初始化列表为空
SequentialList() : head(nullptr) {}
// 插入元素到列表头部
void insertAtFront(int value) {
ListNode newNode{value, head};
head = &newNode;
}
// 插入元素到列表尾部
void insertAtEnd(int value) {
if (!head) {
head = new ListNode(value);
return;
}
ListNode* current = head;
while (current->next) {
current = current->next;
}
current->next = new ListNode(value);
}
// 删除指定值的第一个出现
void remove(int value) {
if (!head || head->data == value) {
ListNode temp = head;
head = head->next;
delete temp;
return;
}
ListNode* current = head;
while (current->next && current->next->data != value) {
current = current->next;
}
if (current->next) {
ListNode* temp = current->next;
current->next = current->next->next;
delete temp;
}
}
// 查找并返回指定值的位置(如果没有找到返回 -1)
int find(int value) {
ListNode* current = head;
for (int i = 0; current && current->data != value; i++, current = current->next) {
if (i >= MAX_SIZE) { // 如果超过最大大小,则返回 -1 表示未找到
return -1;
}
}
return current ? i : -1; // 返回索引,如果找到则非空,否则返回 -1
}
// 显示整个列表
void display() {
ListNode* current = head;
while (current) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << "\n";
}
private:
ListNode* head; // 链表头结点
};
// 程序入口,创建实例并进行相应操作
int main() {
SequentialList list;
// 使用list对象调用上述方法...
}
```
相关推荐
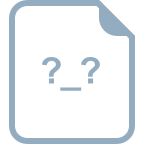
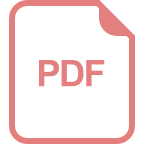













