计算1!+2!+3!+…+10!。分别用单循环结构和双重循环结构。用Long注释
时间: 2024-12-22 08:22:12 浏览: 2
计算阶乘之和1! + 2! + 3! + ... + 10!,我们可以采用递归或循环的方式实现。这里先给出基本的数学公式:n! = n * (n - 1)!。
**使用单循环结构(递归实现):**
由于阶乘的计算涉及到递归,不适合用单纯的循环,但我们可以用递归来实现并记录每个阶乘的结果,然后求和。因为Java的`long`类型可以存储较大的数值,我们加上`@Override`和`@SafeVarargs`注解来避免无限递归:
```java
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
public @interface SafeVarargs {
}
public class FactorialSum {
@SafeVarargs
public static long factorialSum(int... numbers) {
return calculateFactorialAndSum(numbers);
}
private static long calculateFactorialAndSum(int[] numbers) {
long sum = 0;
for (int num : numbers) {
sum += factorial(num);
}
return sum;
}
private static long factorial(int n) {
if (n == 1 || n == 0) {
return 1;
} else {
// 为了防止溢出,使用long类型
return (long)n * factorial(n - 1); // 使用递归
}
}
public static void main(String[] args) {
System.out.println(factorialSum(1, 2, 3, 4, 5, 6, 7, 8, 9, 10));
}
}
```
**使用双层循环结构(迭代实现):**
在这种情况下,我们可以创建两个嵌套循环来逐个计算阶乘,并将结果累加。同样需要注意的是,为了避免整数溢出,需要使用`long`类型:
```java
public class IterativeFactorialSum {
public static long iterativeFactorialSum() {
long sum = 0;
for (int i = 1; i <= 10; i++) {
long fact = 1;
for (int j = 2; j <= i; j++) {
fact *= j;
}
sum += fact;
}
return sum;
}
public static void main(String[] args) {
System.out.println(iterativeFactorialSum());
}
}
```
阅读全文
相关推荐
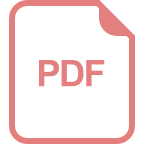
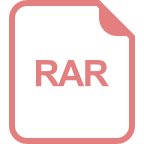
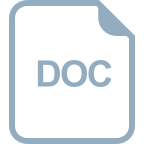
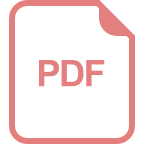
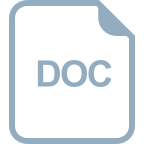
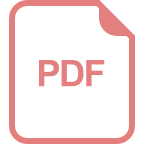
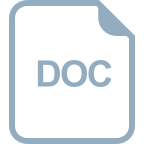
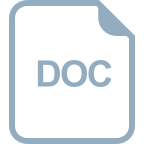
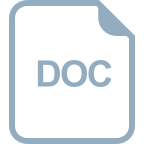
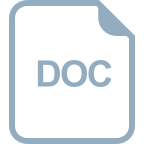
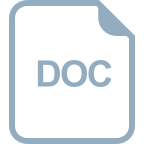
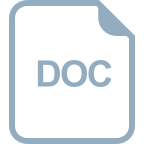
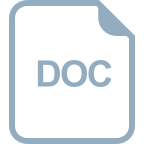
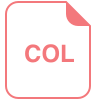
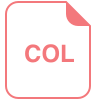
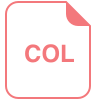
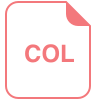
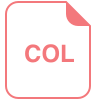
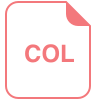