redis 分布式锁 java代码 spring boot
时间: 2023-07-31 21:13:52 浏览: 126
在 Spring Boot 中使用 Redis 实现分布式锁,你可以按照以下步骤进行:
1. 添加 Redis 依赖
在 `pom.xml` 文件中添加 Redis 相关的依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
```
2. 配置 Redis 连接
在 `application.properties` 或 `application.yml` 文件中配置 Redis 连接信息:
```properties
spring.redis.host=127.0.0.1
spring.redis.port=6379
```
3. 创建分布式锁工具类
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.stereotype.Component;
import java.util.concurrent.TimeUnit;
@Component
public class RedisDistributedLock {
@Autowired
private RedisTemplate<String, String> redisTemplate;
public boolean lock(String lockKey, String requestId, long expireTime) {
Boolean success = redisTemplate.opsForValue().setIfAbsent(lockKey, requestId, expireTime, TimeUnit.MILLISECONDS);
return success != null && success;
}
public boolean releaseLock(String lockKey, String requestId) {
String value = redisTemplate.opsForValue().get(lockKey);
if (value != null && value.equals(requestId)) {
return redisTemplate.delete(lockKey);
}
return false;
}
}
```
4. 在需要加锁的地方使用分布式锁
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/example")
public class ExampleController {
@Autowired
private RedisDistributedLock distributedLock;
@GetMapping("/lock")
public String lockExample() {
String lockKey = "exampleLock";
String requestId = UUID.randomUUID().toString();
long expireTime = 5000; // 锁的过期时间,单位为毫秒
// 尝试获取锁
boolean lockSuccess = distributedLock.lock(lockKey, requestId, expireTime);
if (lockSuccess) {
try {
// 执行业务逻辑
Thread.sleep(2000);
return "Success";
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
// 释放锁
distributedLock.releaseLock(lockKey, requestId);
}
}
return "Failed";
}
}
```
在上述代码中,首先创建了一个 `RedisDistributedLock` 的工具类,用来进行锁的获取和释放操作。然后,在需要加锁的地方调用 `lock()` 方法尝试获取锁,如果获取成功,则执行业务逻辑;最后,在业务逻辑执行完成后,调用 `releaseLock()` 方法释放锁。
注意:在上述示例中,使用了 `RedisTemplate` 作为 Redis 的操作模板,你可以根据实际情况进行调整和优化。另外,还可以对分布式锁进行进一步的优化,例如使用 Lua 脚本实现原子性操作等。
阅读全文
相关推荐
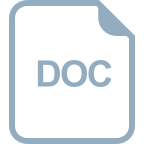
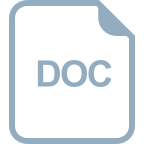
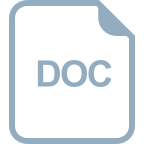

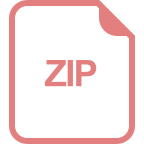
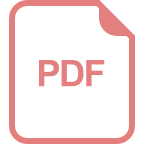
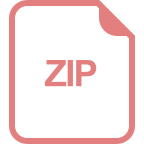
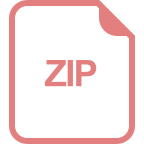
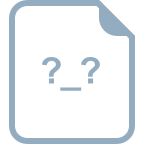








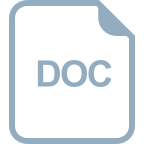