优化这段代码#include <stdio.h> #include <string.h> #include <sys/types.h> /* See NOTES */ #include <sys/socket.h> #include <netinet/in.h> #include <stdlib.h> #include <arpa/inet.h> #include <unistd.h> void *Writhdata(void *arge) { int ret=((void*)arge); char buf[32]={0}; while(1) { if(read(ret,buf,32)>0) { printf("buf=%s\r\n",buf); memset(buf,0,32); } } } int main(int argc,char *argv[]) { int sockfd; struct sockaddr_in cli; int port; int ret; pthread_t writethread; int running2=1; char buf[32]={0}; int str[32]={0}; sockfd =socket(AF_INET,SOCK_STREAM,0); if(sockfd==-1) { perror("socket is fail\r\n"); } port=atoi(argv[2]); cli.sin_addr.s_addr=inet_addr(argv[1]); cli.sin_family=AF_INET; cli.sin_port=htons(port); ret=connect(sockfd,(struct sockaddr *)&cli,sizeof(struct sockaddr_in)); //客户端链接服务器 if(ret==-1) { perror("connect is fail\r\n"); } pthread_create(&writethread,NULL,Writhdata,&ret); while(running2) { fgets (str,32,stdin); if(strstr(str,"exit") != NULL) { running2=0; write(ret,"c-bay!!!",9); } write(ret,str,32); } }
时间: 2024-04-15 09:26:24 浏览: 29
这段代码可以进行一些优化和改进。以下是一些可能的优化建议:
1. 错误处理:在代码中添加适当的错误处理机制,例如对`socket()`、`connect()`函数的返回值进行检查,并在出错时进行相应的处理,如打印错误信息并退出程序。
2. 使用`const`修饰参数:在`main()`函数中的`char *argv[]`参数可以使用`const`修饰,因为这些参数不会被修改。
3. 使用`sizeof`获取数组大小:在使用`memset()`函数时,可以使用`sizeof`操作符获取数组的大小,而不是手动指定32。
4. 避免多次重复设置数组为0:在`Writhdata()`函数中,可以将`memset(buf, 0, sizeof(buf));`移动到while循环外部,在每次接收数据之前只需清空一次。
5. 优化接收数据:可以使用`recv()`函数代替`read()`函数来接收数据,并根据返回值判断接收是否成功,避免因为接收失败而造成的无限循环。
6. 优化发送数据:在循环中使用`write()`发送数据时,应该将要发送的数据长度作为第三个参数传递给`write()`函数,而不是固定地传递32。
7. 线程安全:在多线程环境中,对共享资源(如标准输出)的访问应该进行同步处理,以避免竞态条件。可以使用互斥锁(例如`pthread_mutex_t`)来保护共享资源的访问。
这些只是一些建议,具体的优化策略还需要根据实际需求和场景进行调整。希望对你有帮助!如果有任何进一步的问题,请随时提问。
相关问题
include <stdio.h> #include <string.h
这两个预处理指令(#include)在C语言中是非常常见的,它们用于引入标准库中的头文件。`<stdio.h>` 是标准输入输出头文件,提供了基本的输入输出功能,如 printf() 和 scanf() 函数,用于控制台的输入输出操作。
`<string.h>` 则是字符串处理的头文件,提供了字符串操作相关的函数,比如 strlen() 用于计算字符串长度,strcpy() 和 strncpy() 用于字符串复制等。
当你在C或C++程序的顶部使用 `#include <stdio.h>` 和 `#include <string.h>`,编译器会查找这些文件并把其中定义的函数原型(函数签名)添加到你的程序中,这样你就可以直接调用这些函数而无需关心它们的具体实现细节。
只使用#include <stdio.h> #include <string.h>
这两个头文件都是C语言标准库中的头文件,其中<stdio.h>包含了一些输入输出函数的声明,如printf和scanf;<string.h>包含了一些字符串操作函数的声明,如strcpy和strlen。使用这两个头文件可以完成很多基本的C语言程序设计,例如输出、输入、字符串操作等等。下面给出一个简单的例子:
```
#include <stdio.h>
#include <string.h>
int main() {
char str1[10] = "hello";
char str2[10] = "world";
printf("%s ", str1);
printf("%s\n", str2);
strcat(str1, str2);
printf("%s\n", str1);
printf("The length of str1 is %d.\n", strlen(str1));
return 0;
}
```
这个程序输出了两个字符串"hello"和"world",然后将它们拼接在一起并输出结果"helloworld",最后输出了拼接后的字符串长度11。
相关推荐
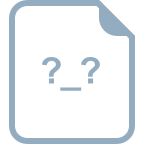
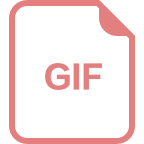
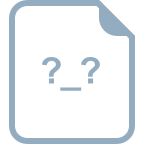













