#include <pthread.h> #include <stdio.h> #include <stdlib.h> #include <string.h> #include "MQTTClient.h" #include <stdint.h> #include <signal.h> /* sigaction */ #include <errno.h> #include "sqlite3.h" #include "pan_reg.h" #include "spi_func.h" #include "auxiliary.h" #if !defined(WIN32) #include <unistd.h> #else #include <windows.h> #endif #include <netdb.h> #include <sys/types.h> #include <sys/socket.h> #include <netinet/in.h> #include <math.h> /* modf */ #include "cJSON.h" #include <fcntl.h> #include "uart.h" #include <sys/types.h> #include <sys/ipc.h> #include <sys/msg.h>
时间: 2023-08-15 10:05:46 浏览: 80
这是一段 C 语言的代码,包含了一些头文件的引用,如 pthread.h、stdio.h、stdlib.h、string.h、MQTTClient.h、stdint.h、signal.h、errno.h、sqlite3.h、netdb.h、sys/types.h、sys/socket.h、netinet/in.h、math.h、cJSON.h、fcntl.h 和 uart.h。这段代码中可能包含了一些线程库、网络库、消息队列、JSON 解析等相关的函数。具体实现需要查看代码的具体逻辑。
相关问题
补全代码#include <stdio.h> #include <stdlib.h> #include <string.h> #include <errno.h> #include <unistd.h> #include <sys/types.h> #include <sys/socket.h> #include <sys/shm.h> #include <pthread.h> #include "mypthread.h" #include "serial.h" #include "zigbee.h" #include "my_camera.h" #include "server.h" static int zgbfd; static pthread_mutex_t cam_mutex; void *pthread_cam(void *arg) { } void *pthread_zgb(void *arg) { } void *pthread_cli(void *arg) { }
下面是对代码的补全:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <errno.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <sys/shm.h>
#include <pthread.h>
#include "mypthread.h"
#include "serial.h"
#include "zigbee.h"
#include "my_camera.h"
#include "server.h"
static int zgbfd;
static pthread_mutex_t cam_mutex;
void *pthread_cam(void *arg)
{
// 摄像头相关操作的具体实现
while (1) {
// 在这里实现摄像头的操作逻辑
// 例如获取摄像头图像并处理
// 使用互斥锁保护临界区
pthread_mutex_lock(&cam_mutex);
// 执行摄像头操作
pthread_mutex_unlock(&cam_mutex);
// 休眠一段时间
usleep(1000);
}
return NULL;
}
void *pthread_zgb(void *arg)
{
// Zigbee 相关操作的具体实现
while (1) {
// 在这里实现 Zigbee 的操作逻辑
// 例如发送或接收数据
// 使用互斥锁保护临界区
pthread_mutex_lock(&cam_mutex);
// 执行 Zigbee 操作
pthread_mutex_unlock(&cam_mutex);
// 休眠一段时间
usleep(1000);
}
return NULL;
}
void *pthread_cli(void *arg)
{
// 客户端连接和通信操作的具体实现
int client_sock = *((int*)arg);
char buffer[1024];
while (1) {
// 在这里实现客户端连接和通信的操作逻辑
// 例如接收客户端消息并处理
memset(buffer, 0, sizeof(buffer));
int ret = read(client_sock, buffer, sizeof(buffer));
if (ret <= 0) {
// 客户端断开连接或出错,结束线程
close(client_sock);
break;
}
// 处理客户端消息
}
return NULL;
}
```
以上补全了 `pthread_cam`、`pthread_zgb` 和 `pthread_cli` 函数的代码实现部分。你可以根据具体需求在这些函数中添加逻辑来完成相应的操作。注意使用互斥锁来保护临界区,以避免多个线程同时访问造成的竞争条件。
解释以下头文件:#include <stdio.h> #include <stdlib.h> #include <stdint.h> #include <string.h> #include <unistd.h> #include <fcntl.h> #include <pthread.h> #include <sys/types.h> #include <sys/socket.h> #include <netinet/in.h> #include <ctype.h> #include <sys/pps.h> #include <sys/types.h> #include "camera.h" #include <encoder.h> #include "ppsFunc.h" #include "timeDiff.h"
这些头文件是C语言程序中常用的系统库和自定义头文件。下面对它们进行简要的解释:
- stdio.h:输入输出相关的函数库,如printf、scanf等。
- stdlib.h:标准库函数,包括各种常用函数,如malloc、free等。
- stdint.h:定义了一些整数类型,如int8_t、uint16_t等。
- string.h:提供字符串操作相关的函数,如strcpy、strcat等。
- unistd.h:提供对POSIX操作系统API的访问,如close、read等。
- fcntl.h:提供对文件控制相关的函数,如open、fcntl等。
- pthread.h:多线程相关的函数库,如pthread_create、pthread_join等。
- sys/types.h:提供对系统数据类型的定义,如pid_t、mode_t等。
- sys/socket.h:提供对套接字的操作,如socket、bind等。
- netinet/in.h:提供对TCP/IP网络编程的支持,如struct sockaddr_in等。
- ctype.h:提供对字符分类和转换的函数,如isalpha、isdigit等。
- sys/pps.h:提供对PPS(Pulse-Per-Second)信号的读取和控制。
- camera.h:自定义头文件,可能是和摄像头相关的函数和数据结构。
- encoder.h:自定义头文件,可能是和视频编码相关的函数和数据结构。
- ppsFunc.h:自定义头文件,可能是和PPS信号相关的函数和数据结构。
- timeDiff.h:自定义头文件,可能是和时间差相关的函数和数据结构。
相关推荐
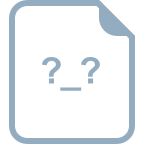
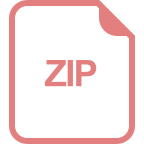
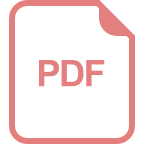













